Java has 3 ways to execute loops
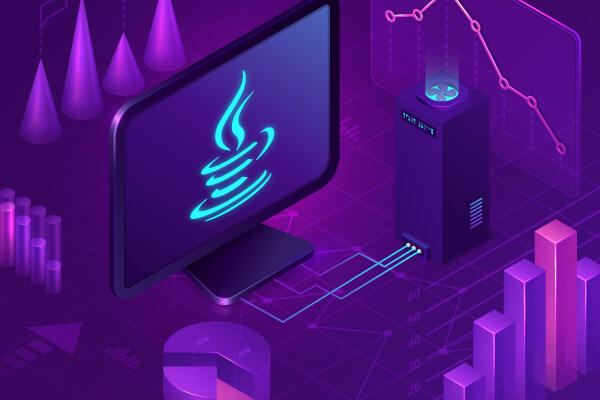
When the programmer wants to execute a set of the program repeatedly until some condition can use the looping concept in java. Java has 3 ways to execute loops.
1.While loop
2.Do…while loop
3.For loop
Though their purpose is the same syntax is different for them.
1. While loop:
In the while loop, until the condition is true code inside the loop will be executed. The syntax for the while loop is as follows.
while(condition){
statements;
}
Steps:
1. Checking the condition, if it’s true then executing the statements inside the loop.
2. Once all the statements are executed inside the loop cursor comes back at the start of the while loop and checks the condition again.
3. When the condition is false loop terminates and the cursor will proceed with the next statements outside the loop if any.
Flow diagram:
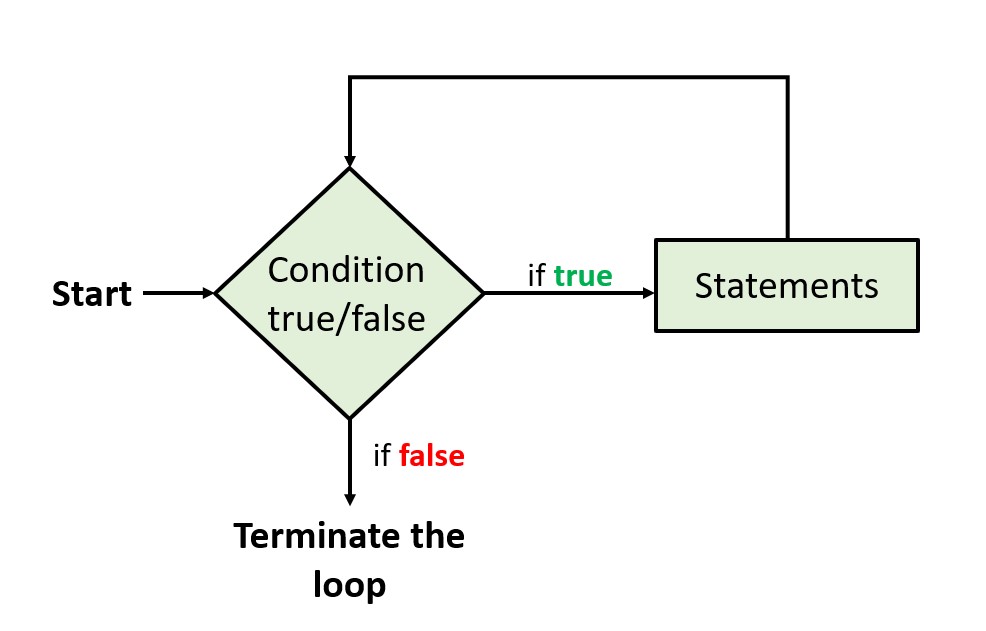
2. Do…while loop
In the do…while loop first statements inside the loop will be printed and then the condition is checked. The syntax for the do…while loop is as follows.
do{
statements;
}while(condition);
Steps:
1. First-time statements inside the loop will be executed before checking the condition. Hence no matter even if the condition is true or false statements inside do…while will be executed at least once.
2. After executing the code inside the do loop cursor comes to the while condition. Until the condition is true, statements inside the loop will be executed.
3. Once the condition is false loop will be terminated and the cursor will proceed with further code outside the loop if any.
Flow chart:
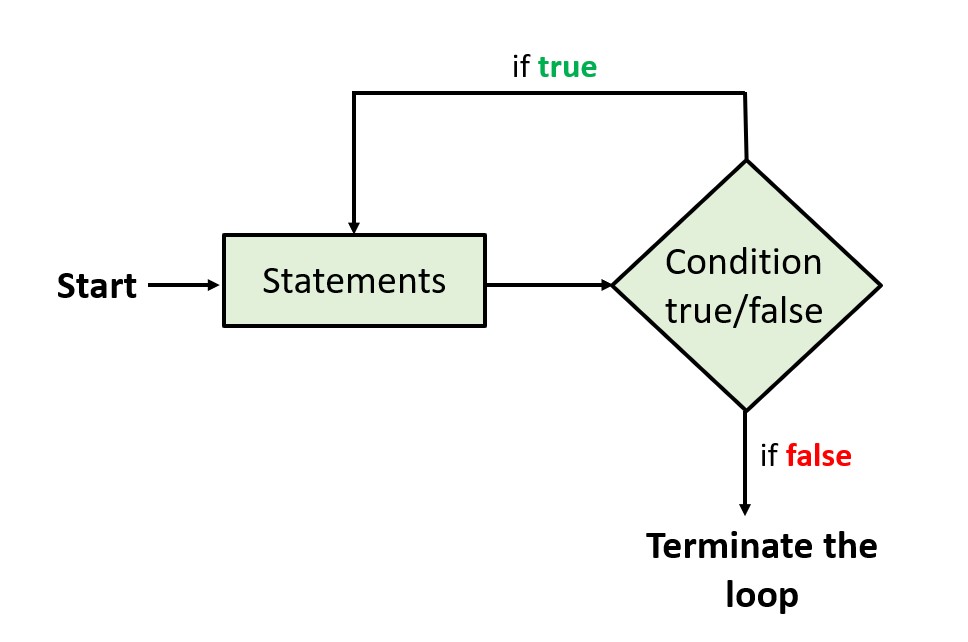
3. For loop:
For loop has variable declared and initialized, condition checking and incrementing/decrementing the variable. The syntax for the for loop is as follows.
for(initialization variable; true/false condition; increment/decrement){
Statements;
}
Steps:
1. The first step for the for loop is to initialize the variable.
2. Once variable is initialized then checking if that condition is true or false.
3. If condition is true then statements inside the loop will get executed.
4. Once all the statements inside the loop are executed then incrementing/decrementing the variable value.
5. After incrementing/decrementing will again check the condition is true/false and loop will continue until condition is false.
Flow diagram:
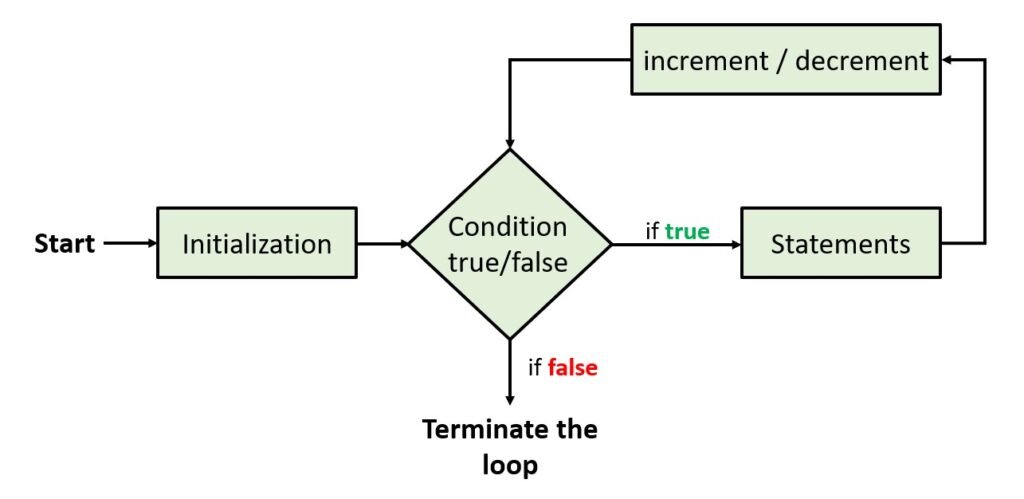
Enhanced for loop:
We can also use another for loop syntax instead of above loop. This type is also called as for-each loop.
Syntax for the for-each loop is as follows.
for(Object a : Collection/Array){
statements;
}
This loop is used to iterate over object of Collection class or array. In the for-each loop value of same array or object on which loop is iterating can not be modified. Hence object used inside for-each condition is immutable.
Note that if we want to use index of array or collection object then better to use general for loop instead of for-each loop.
From java-8 onwards this for-each is further simplified as follows.
collection.forEach(a -> {
statements;
});
Following is a comparison between the for-each loop and java-8 forEach loop:
