Taking input from the user
From java, we can take the user’s entered output from the console. There are four types to get user’s input from the user.
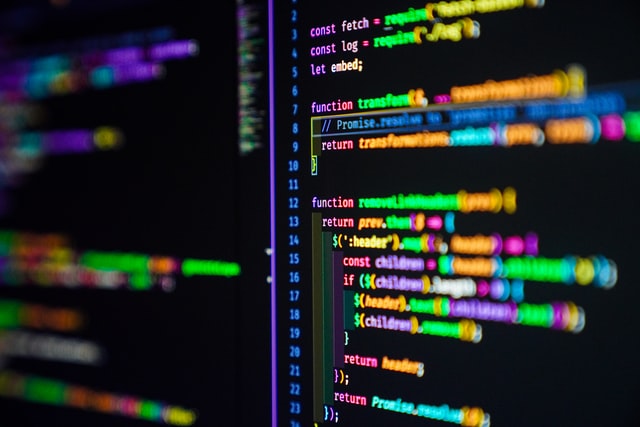
1. Using Buffered Reader class
This is introduced in the JDK1.0 version, an example for buffered reader class is as shown. BufferedReder class is from the java.io package. To read other types like integer we can cast it using Integer.parseInt() method.
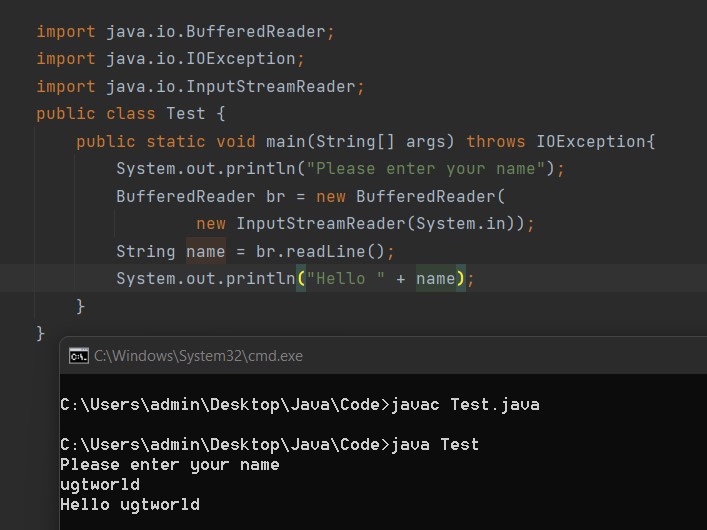
Following are different methods in BufferedReader class.
Method | Description |
void close() | This closes the input stream and releases the resources. |
Stream<String> lines() | Returns a Stream, the elements of which are lines read from this BufferedReader. |
void mark(int readAheadLimit) | Marks the current position in the stream. |
boolean markSupported() | Tells whether this stream supports the mark() operation. |
int read() | Used for reading single character. |
int read(char[] charArr, int off, int len) | Reads characters into a portion of an array. |
String readLine() | Reads a line of text. |
boolean ready() | Checks whether this stream is ready to be read. |
void reset() | Resets the stream to the most recent mark. |
long skip(long n) | Take a number n and skip that many characters |
2. Using Scanner class
The scanner class is from java.util package. An example to read data using the scanner class is as shown below.
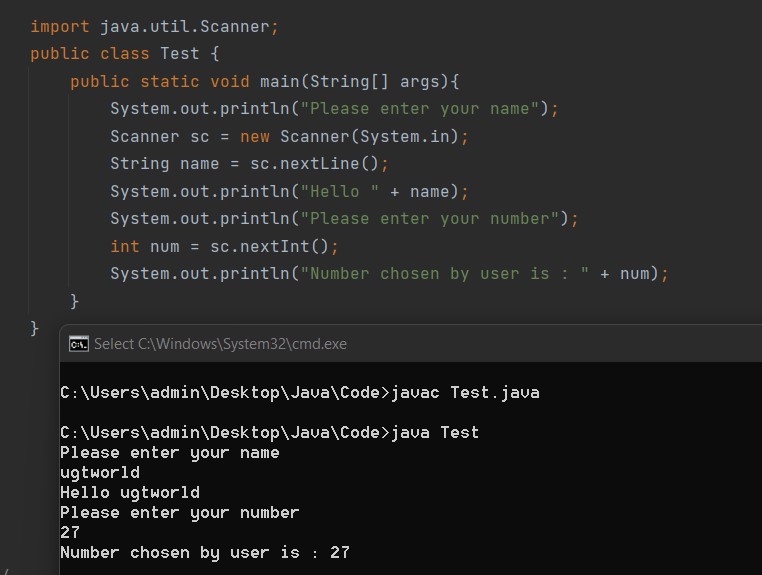
Following are different methods in the Scanner class to read different types of inputs :
Method | Description |
boolean nextBoolean() | To read a boolean value from the user. |
byte nextByte() | To read a byte value from the user. |
double nextDouble() | To read a double value from the user. |
float nextFloat() | To read a float value from the user. |
int nextInt() | To read an int value from the user. |
String nextLine() | To read a String value from the user. |
long nextLong() | To read a long value from the user. |
short nextShort() | To read a short value from the user. |
Scanner class also provides some different methods other than above we can find them on
https://docs.oracle.com/javase/8/docs/api/java/util/Scanner.html .
3. Using command-line arguments
This is commonly used to read the input from users. This is passed while executing the code and we can read it from a string array input param from the main method. Values are split by space. If we want to add a string with space we can add it as “Welcome user”.
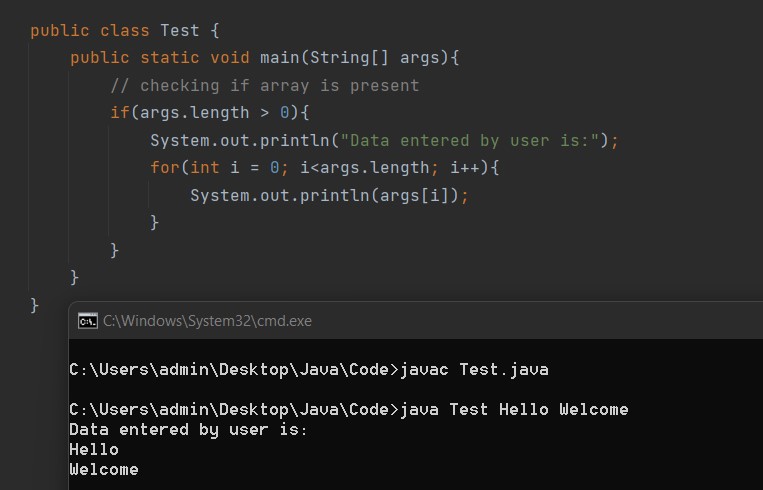
4. Using Console class
System class’s console() method returns the object of the Console class. The “readLine” method is used to read the input from a user.
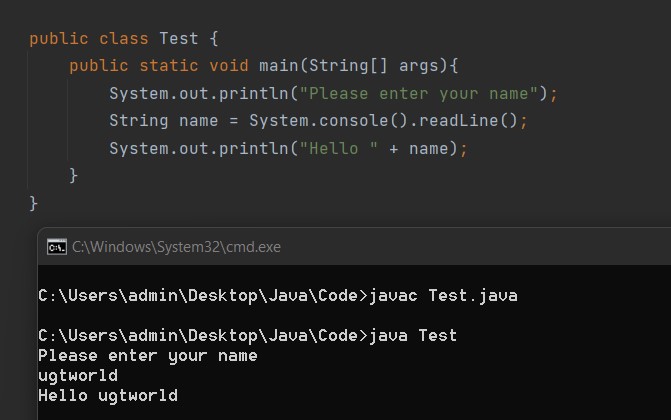
Following are the different methods for the Console class:
Method | Description |
void flush() | Flushes the console and forces any buffered output to be written immediately. |
Console format(String fmt, Object… args) | Writes a formatted string to the console’s output stream. |
Console printf(String format, Object… args) | A convenient method to write a formatted string to the console’s output. |
Reader reader() | It is used to retrieve the unique reader object associated with the console. |
String readLine() | Reads a single line of text from the console. |
String readLine(String fmt, Object… args) | To get formatted prompt and then reads a single line of text from the console. |
char[] readPassword() | Reads a password from the console with echoing disabled. |
char[] readPassword(String fmt, Object… args) | To get a formatted prompt then reads a password from the console with echoing disabled. |
PrintWriter writer() | To get the unique PrintWriter object associated with this console. |