LinkedHashMap in Java is a child class of HashMap, It’s internally implemented using HashTable and LinkedList.
We have seen HashMap in Java Since HashMap is not able to maintain the insertion order but when we want to maintain the insertion order then we can use LinkedHashMap in Java instead of HashMap.
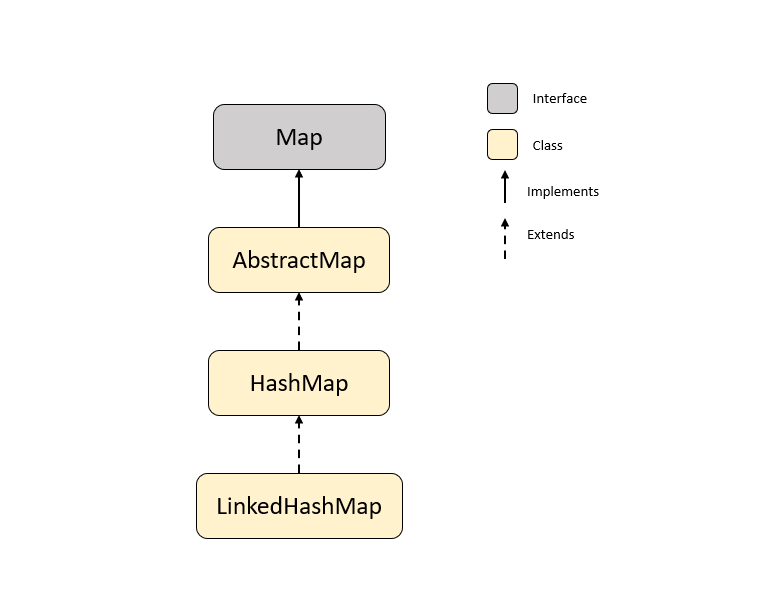
It is exactly the same as the HashMap except for the following differences.
HashMap | LinkedHashMap |
The underlying data structure is HashTable | The underlying data structure is LinkedList and HashTable |
Insertion order is not preserved and it is based on a hash code of keys. | Insertion order is preserved. |
Introduced in 1.2v | Introduced in 1.4v |
Following are the constructors of LinkedHashMap.
LinkedHashMap has the same constructors as HashMap including one new constructor having accessOrder as a parameter.
- public LinkedHashMap(): Creates an empty insertion-ordered LinkedHashMap instance with the default initial capacity (16) and load factor (0.75).
- public LinkedHashMap(int initialCapacity): Creates an empty insertion-ordered LinkedHashMap instance with the specified initial capacity and a default load factor (0.75).
- public LinkedHashMap(int initialCapacity, float loadFactor): Creates an empty insertion-ordered LinkedHashMap instance with the specified initial capacity and load factor.
- public LinkedHashMap(Map<? extends K,? extends V> m): Creates an insertion-ordered LinkedHashMap instance with the same mappings as the specified map.
- public LinkedHashMap(int initialCapacity, float loadFactor, boolean accessOrder): Creates an empty LinkedHashMap instance with the specified initial capacity, load factor, and ordering mode. (if accessOrder is true then the keys are sorted from least recently accessed to most recently accessed).
Following is the example for the above 5 constructors.
import java.util.LinkedHashMap;
class Test {
public static void main(String[] args) {
// 1. By Using public LinkedHashMap()
LinkedHashMap<Integer, String> linkedMap1 = new LinkedHashMap();
linkedMap1.put(1, “EGHJ”);
linkedMap1.put(100, “EGHP”);
System.out.println(“linkedMap1: “+ linkedMap1);
// 2. By Using public LinkedHashMap(int initialCapacity)
LinkedHashMap<Integer, String> linkedMap2 = new LinkedHashMap(20);
linkedMap2.put(14, “HHDG”);
linkedMap2.put(74, “GDHJ”);
System.out.println(“linkedMap2: “+ linkedMap2);
// 3. By Using public LinkedHashMap(int initialCapacity, float loadFactor)
LinkedHashMap<Integer, String> linkedMap3 = new LinkedHashMap(20, 0.5f);
linkedMap3.put(5, “OPRY”);
linkedMap3.put(12, “OPQR”);
System.out.println(“linkedMap3: “+ linkedMap3);
// 4. By Using public LinkedHashMapLinkedHashMap(Map<? extends K,? extends V> m)
LinkedHashMap<Integer, String> linkedMap4 = new LinkedHashMap(linkedMap1);
linkedMap4.put(10, “LAYP”);
linkedMap4.put(88, “KAIL”);
System.out.println(“linkedMap4: “+ linkedMap4);
// 5. By using public LinkedHashMap(int initialCapacity, float loadFactor, boolean accessOrder)
LinkedHashMap<Integer, String> linkedMap5 = new LinkedHashMap(10, 0.75f, true);
linkedMap5.put(34,”KSJN”);
linkedMap5.put(65,”BHCN”);
linkedMap5.put(23,”SEKA”);
linkedMap5.put(21,”JKVB”);
linkedMap5.put(98,”UPWV”);
linkedMap5.put(45,”RMSG”);
System.out.println(“linkedMap5 Before accessing values: “+ linkedMap5);
System.out.println(“accessing key 98 “+ linkedMap5.get(98));
System.out.println(“accessing key 65 “+ linkedMap5.get(65));
System.out.println(“accessing key 21 “+ linkedMap5.get(21));
// From the output based on accessed order entries for key 98, 65 and 21 are rearranged.
System.out.println(“linkedMap5 after accessing value: “+ linkedMap5);
}
}
class Test {
public static void main(String[] args) {
// 1. By Using public LinkedHashMap()
LinkedHashMap<Integer, String> linkedMap1 = new LinkedHashMap();
linkedMap1.put(1, “EGHJ”);
linkedMap1.put(100, “EGHP”);
System.out.println(“linkedMap1: “+ linkedMap1);
// 2. By Using public LinkedHashMap(int initialCapacity)
LinkedHashMap<Integer, String> linkedMap2 = new LinkedHashMap(20);
linkedMap2.put(14, “HHDG”);
linkedMap2.put(74, “GDHJ”);
System.out.println(“linkedMap2: “+ linkedMap2);
// 3. By Using public LinkedHashMap(int initialCapacity, float loadFactor)
LinkedHashMap<Integer, String> linkedMap3 = new LinkedHashMap(20, 0.5f);
linkedMap3.put(5, “OPRY”);
linkedMap3.put(12, “OPQR”);
System.out.println(“linkedMap3: “+ linkedMap3);
// 4. By Using public LinkedHashMapLinkedHashMap(Map<? extends K,? extends V> m)
LinkedHashMap<Integer, String> linkedMap4 = new LinkedHashMap(linkedMap1);
linkedMap4.put(10, “LAYP”);
linkedMap4.put(88, “KAIL”);
System.out.println(“linkedMap4: “+ linkedMap4);
// 5. By using public LinkedHashMap(int initialCapacity, float loadFactor, boolean accessOrder)
LinkedHashMap<Integer, String> linkedMap5 = new LinkedHashMap(10, 0.75f, true);
linkedMap5.put(34,”KSJN”);
linkedMap5.put(65,”BHCN”);
linkedMap5.put(23,”SEKA”);
linkedMap5.put(21,”JKVB”);
linkedMap5.put(98,”UPWV”);
linkedMap5.put(45,”RMSG”);
System.out.println(“linkedMap5 Before accessing values: “+ linkedMap5);
System.out.println(“accessing key 98 “+ linkedMap5.get(98));
System.out.println(“accessing key 65 “+ linkedMap5.get(65));
System.out.println(“accessing key 21 “+ linkedMap5.get(21));
// From the output based on accessed order entries for key 98, 65 and 21 are rearranged.
System.out.println(“linkedMap5 after accessing value: “+ linkedMap5);
}
}
Output:
linkedMap1: {1=EGHJ, 100=EGHP}linkedMap2: {14=HHDG, 74=GDHJ}
linkedMap3: {5=OPRY, 12=OPQR}
linkedMap4: {1=EGHJ, 100=EGHP, 10=LAYP, 88=KAIL}
linkedMap5 Before accessing values: {34=KSJN, 65=BHCN, 23=SEKA, 21=JKVB, 98=UPWV, 45=RMSG}
accessing key 98 UPWV
accessing key 65 BHCN
accessing key 21 JKVB
linkedMap5 after accessing value: {34=KSJN, 23=SEKA, 45=RMSG, 98=UPWV, 65=BHCN, 21=JKVB}
LinkedHashMap has the same methods as in HashMap, we can refer to different types of methods from HashMap in Java.
LinkedHashMap has one extra method as below.
- protected boolean removeEldestEntry(Map.Entry<K,V> eldest): Returns true if this map should remove its eldest entry.
import java.util.LinkedHashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
final int MAX_ENTRIES = 3;
LinkedHashMap<Integer, String> linkedMap = new LinkedHashMap(MAX_ENTRIES, 0.75f){
protected boolean removeEldestEntry(Map.Entry eldest) {
// if size is > 3 eldest entry will be removed
return size() > MAX_ENTRIES;
}
};
linkedMap.put(10, “ABCD10”);
linkedMap.put(33, “ABCD33”);
linkedMap.put(20, “ABCD20”);
System.out.println(“Original map: ” + linkedMap);
// if we add another entry then eldest entry i.e for key 10 will be removed
linkedMap.put(18, “ABCD18”);
System.out.println(“Updated map : ” + linkedMap);
// if we add another entry then eldest entry i.e for key 33 will be removed
linkedMap.put(11, “ABCD11”);
System.out.println(“Final map : ” + linkedMap);
}
}
import java.util.Map;
public class Main {
public static void main(String[] args) {
final int MAX_ENTRIES = 3;
LinkedHashMap<Integer, String> linkedMap = new LinkedHashMap(MAX_ENTRIES, 0.75f){
protected boolean removeEldestEntry(Map.Entry eldest) {
// if size is > 3 eldest entry will be removed
return size() > MAX_ENTRIES;
}
};
linkedMap.put(10, “ABCD10”);
linkedMap.put(33, “ABCD33”);
linkedMap.put(20, “ABCD20”);
System.out.println(“Original map: ” + linkedMap);
// if we add another entry then eldest entry i.e for key 10 will be removed
linkedMap.put(18, “ABCD18”);
System.out.println(“Updated map : ” + linkedMap);
// if we add another entry then eldest entry i.e for key 33 will be removed
linkedMap.put(11, “ABCD11”);
System.out.println(“Final map : ” + linkedMap);
}
}
Output:
Original map: {10=ABCD10, 33=ABCD33, 20=ABCD20}Updated map : {33=ABCD33, 20=ABCD20, 18=ABCD18}
Final map : {20=ABCD20, 18=ABCD18, 11=ABCD11}