IdentityHashMap in Java implements Map interface and extends AbstractMap.
IdentityHashMap in Java also implements a Map interface using HashTable. This Class is used when the user wants to compare the objects via reference instead of equals().
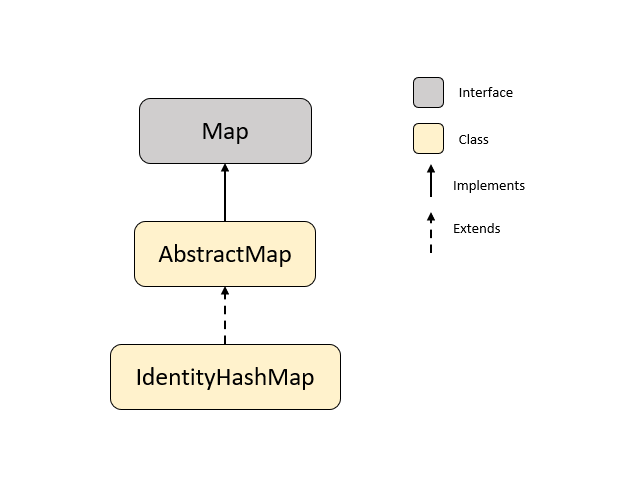
IdentityHashMap is also the same as HashMap in Java except for the following difference.
In the case of normal HashMap, JVM will use the ‘.equals()’ method to identify duplicate keys, which is meant for content comparison.
But In the Case of IdentityHashMap JVM will use the ‘==’ operator to identify duplicate keys. Which is meant for reference comparison (address comparison).
Let’s check the following example for the above points.
import java.util.IdentityHashMap;
class Test {
public static void main(String[] args) {
Integer i1 = new Integer(10);
Integer i2 = new Integer(10);
HashMap map = new HashMap();
map.put(i1,”A”);
map.put(i2,”B”);
System.out.println(“HashMap: ” + map);
IdentityHashMap identityMap = new IdentityHashMap();
identityMap.put(i1,”A”);
identityMap.put(i2,”B”);
System.out.println(“IdentityHashMap: ” + identityMap);
}
}
IdentityHashMap: {10=A, 10=B}
Following are the constructors used in IdentityHashMap:
- public IdentityHashMap(): Creates a new, empty identity hash map with a default expected maximum size (21).
- public IdentityHashMap(int expectedMaxSize): Creates a new, empty map with the specified expected maximum size.
- public IdentityHashMap(Map<? extends K,? extends V> m): Creates a new identity hash map containing the keys-value mappings in the specified map.
Following is example for above 3 constructors.
class Test {
public static void main(String[] args) {
// Using IdentityHashMap()
IdentityHashMap map1 = new IdentityHashMap();
map1.put(21,”CHHN”);
map1.put(88,”KAIL”);
System.out.println(“map1: ” + map1);
// Using IdentityHashMap(int expectedMaxSize)
IdentityHashMap map2 = new IdentityHashMap(25);
map2.put(2,”GHAT”);
map2.put(8,”LJDT”);
map2.put(12,”LDFJ”);
System.out.println(“map2: ” + map2);
// Using IdentityHashMap(Map<? extends K,? extends V> m)
IdentityHashMap map3 = new IdentityHashMap(map1);
map3.put(43,”CHAN”);
System.out.println(“map3: ” + map3);
}
}
map2: {8=LJDT, 12=LDFJ, 2=GHAT}
map3: {21=CHHN, 88=KAIL, 43=CHAN}
Methods in the IdentityHashMap are same as HashMap in Java.
Following are the differences between IdentityHashMap and HashMap.
HashMap | IdentityHashMap |
HashMap uses equals() method is used to compare the keys which means the content comparison. | IdentityHashMap uses ‘==’ operator to compare the keys which means the reference comparison (address comparison). |
HashMap uses hashCode() method to find the bucket location. | IdentityHashMap does not use hashCode() method, instead it uses System.IdentityHashCode() method to find the bucket location. |
Keys in HashMap needs to be immutable | Keys in IdentityHashMap does not required to be immutable. |
Performance is low than IdentityHashMap | Performance is more than HashMap |
Let’s see one more example for IdentityHashMap as follows.
In the following program we can see that str1, str2, str3 are immutable hence when we try to add str1 and str2 into the identity map, only str2 (ABCD) key will get added since IdentityHashMap uses address comparision, and address for str1 and str2 is same.
But for str4, str5, str6, str7 we are creating new objects of string each time hence address values for each if them will be different hence all of these keys will be added in the map.
class Test {
public static void main(String[] args) {
String str1 = “ABCD”;
String str2 = “ABCD”;
String str3 = “DFTF”;
String str4 = new String(“ABCD”);
String str5 = new String(“EFGH”);
String str6 = new String(“EFGH”);
String str7 = new String(“SKLP”);
IdentityHashMap map = new IdentityHashMap();
map.put(str1,”1″);
map.put(str2,”2″);
map.put(str3,”3″);
map.put(str4,”4″);
map.put(str5,”5″);
map.put(str6,”6″);
map.put(str7,”7″);
System.out.println(map);
}
}