An EnumSet in Java is a specialized Set collection for enum classes. It extends from AbstractSet and is available in java.util package.
- All of the elements in an enum set must be of the same enum type that is specified.
- Null elements are not allowed.
- The iterator returned for enum is thread-safe, which means it will not throw ConcurrentModificationException when we try to update the set while iterating.
Since EnumSet in Java is an abstract class we can not create an object of the same. Hence most of the methods present inside the EnumSet class are static.
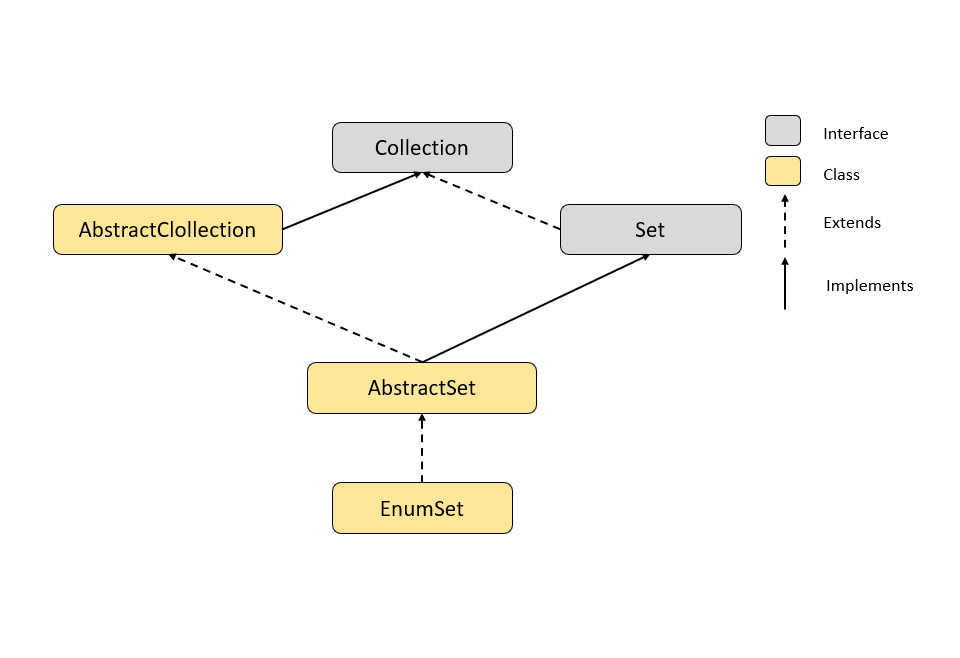
Following are the methods present in EnumSet in Java:
- static <E extends Enum<E>> EnumSet<E> allOf(Class<E> elementType): Creates an enum set containing all of the elements in the specified element type.
- EnumSet<E> clone(): Returns a copy of this set.
- static <E extends Enum<E>> EnumSet<E> complementOf(EnumSet<E> s): Creates an enum set with the same element type as the specified enum set.
- static <E extends Enum<E>> EnumSet<E> copyOf(Collection<E> c): Creates an enum set initialized from the specified collection.
- static <E extends Enum<E>> EnumSet<E> copyOf(EnumSet<E> s): Creates an enum set with the same element type as the specified enum set.
- static <E extends Enum<E>> EnumSet<E> noneOf(Class<E> elementType): Creates an empty enum set with the specified element type.
- static <E extends Enum<E>> EnumSet<E> of(E e): Creates an enum set initially containing the specified element.
- static <E extends Enum<E>> EnumSet<E> of(E first, E… rest): Creates an enum set initially containing the specified elements. E… is an Varargs which means this method takes variable number of arguments i.e. we can pass as many arguments we want of enum type.
- static <E extends Enum<E>> EnumSet<E> of(E e1, E e2): Creates an enum set initially containing the specified elements.
- static <E extends Enum<E>> EnumSet<E> of(E e1, E e2, E e3): Creates an enum set initially containing the specified elements.
- static <E extends Enum<E>> EnumSet<E> of(E e1, E e2, E e3, E e4): Creates an enum set initially containing the specified elements.
- static <E extends Enum<E>> EnumSet<E> of(E e1, E e2, E e3, E e4, E e5): Creates an enum set initially containing the specified elements.
- static <E extends Enum<E>> EnumSet<E> range(E from, E to): Creates an enum set initially containing all of the elements in the range defined by the two specified endpoints.
Following is the example of the above methods in EnumSet in Java:
import java.util.*;
public class Test {
public enum MyEnum {
EDJS, FJEK, SODJ, SEUF, SEF, SERE, DHSF, DFTF, KSOO
}
public static void main(String[] args) {
// 1. Using static <E extends Enum<E>> EnumSet<E> allOf(Class<E> elementType)
EnumSet<MyEnum> set1 = EnumSet.allOf(MyEnum.class);
System.out.println(“Set1 is : ” + set1);
// 2. Using EnumSet<E> clone()
EnumSet<MyEnum> set2 = set1.clone();
System.out.println(“Set2 is : ” + set2);
// 3. Using static <E extends Enum<E>> EnumSet<E> complementOf(EnumSet<E> s)
EnumSet<MyEnum> set3 = EnumSet.complementOf(set1);
System.out.println(“Set3 is : ” + set3);
// 4. Using static <E extends Enum<E>> EnumSet<E> copyOf(Collection<E> c)
List<MyEnum> list = Arrays.asList(MyEnum.FJEK, MyEnum.DFTF, MyEnum.KSOO);
EnumSet<MyEnum> set4 = EnumSet.copyOf(list);
System.out.println(“Set4 is : ” + set4);
// 5. Using static <E extends Enum<E>> EnumSet<E> copyOf(EnumSet<E> s)
EnumSet<MyEnum> set5 = EnumSet.copyOf(set4);
System.out.println(“Set5 is : ” + set5);
// 6. Using static <E extends Enum<E>> EnumSet<E> noneOf(Class<E> elementType)
EnumSet<MyEnum> set6 = EnumSet.noneOf(MyEnum.class);
System.out.println(“Set6 is : ” + set6);
// 7. Using static <E extends Enum<E>> EnumSet<E> of(E e)
EnumSet<MyEnum> set7 = EnumSet.of(MyEnum.DFTF);
System.out.println(“Set7 is : ” + set7);
// 8. Using static <E extends Enum<E>> EnumSet<E> of(E first, E… rest)
EnumSet<MyEnum> set8 = EnumSet.of(MyEnum.DFTF, MyEnum.DHSF, MyEnum.KSOO, MyEnum.SERE, MyEnum.SEF, MyEnum.SEUF,
MyEnum.SODJ);
System.out.println(“Set8 is : ” + set8);
// 9. Using static <E extends Enum<E>> EnumSet<E> of(E e1, E e2)
EnumSet<MyEnum> set9 = EnumSet.of(MyEnum.DFTF, MyEnum.DHSF);
System.out.println(“Set9 is : ” + set9);
// 10. Using static <E extends Enum<E>> EnumSet<E> of(E e1, E e2, E e3)
EnumSet<MyEnum> set10 = EnumSet.of(MyEnum.DFTF, MyEnum.DHSF, MyEnum.EDJS);
System.out.println(“Set10 is : ” + set10);
// 11. Using static <E extends Enum<E>> EnumSet<E> of(E e1, E e2, E e3, E e4)
EnumSet<MyEnum> set11 = EnumSet.of(MyEnum.DFTF, MyEnum.DHSF, MyEnum.EDJS, MyEnum.KSOO);
System.out.println(“Set11 is : ” + set11);
// 12. Using static <E extends Enum<E>> EnumSet<E> of(E e1, E e2, E e3, E e4, E e5)
EnumSet<MyEnum> set12 = EnumSet.of(MyEnum.DFTF, MyEnum.FJEK, MyEnum.DHSF, MyEnum.EDJS, MyEnum.KSOO);
System.out.println(“Set12 is : ” + set12);
// 13. Using static <E extends Enum<E>> EnumSet<E> range(E from, E to)
EnumSet<MyEnum> set13 = EnumSet.range(MyEnum.SODJ, MyEnum.DFTF);
System.out.println(“Set13 is : ” + set13);
}
}
public class Test {
public enum MyEnum {
EDJS, FJEK, SODJ, SEUF, SEF, SERE, DHSF, DFTF, KSOO
}
public static void main(String[] args) {
// 1. Using static <E extends Enum<E>> EnumSet<E> allOf(Class<E> elementType)
EnumSet<MyEnum> set1 = EnumSet.allOf(MyEnum.class);
System.out.println(“Set1 is : ” + set1);
// 2. Using EnumSet<E> clone()
EnumSet<MyEnum> set2 = set1.clone();
System.out.println(“Set2 is : ” + set2);
// 3. Using static <E extends Enum<E>> EnumSet<E> complementOf(EnumSet<E> s)
EnumSet<MyEnum> set3 = EnumSet.complementOf(set1);
System.out.println(“Set3 is : ” + set3);
// 4. Using static <E extends Enum<E>> EnumSet<E> copyOf(Collection<E> c)
List<MyEnum> list = Arrays.asList(MyEnum.FJEK, MyEnum.DFTF, MyEnum.KSOO);
EnumSet<MyEnum> set4 = EnumSet.copyOf(list);
System.out.println(“Set4 is : ” + set4);
// 5. Using static <E extends Enum<E>> EnumSet<E> copyOf(EnumSet<E> s)
EnumSet<MyEnum> set5 = EnumSet.copyOf(set4);
System.out.println(“Set5 is : ” + set5);
// 6. Using static <E extends Enum<E>> EnumSet<E> noneOf(Class<E> elementType)
EnumSet<MyEnum> set6 = EnumSet.noneOf(MyEnum.class);
System.out.println(“Set6 is : ” + set6);
// 7. Using static <E extends Enum<E>> EnumSet<E> of(E e)
EnumSet<MyEnum> set7 = EnumSet.of(MyEnum.DFTF);
System.out.println(“Set7 is : ” + set7);
// 8. Using static <E extends Enum<E>> EnumSet<E> of(E first, E… rest)
EnumSet<MyEnum> set8 = EnumSet.of(MyEnum.DFTF, MyEnum.DHSF, MyEnum.KSOO, MyEnum.SERE, MyEnum.SEF, MyEnum.SEUF,
MyEnum.SODJ);
System.out.println(“Set8 is : ” + set8);
// 9. Using static <E extends Enum<E>> EnumSet<E> of(E e1, E e2)
EnumSet<MyEnum> set9 = EnumSet.of(MyEnum.DFTF, MyEnum.DHSF);
System.out.println(“Set9 is : ” + set9);
// 10. Using static <E extends Enum<E>> EnumSet<E> of(E e1, E e2, E e3)
EnumSet<MyEnum> set10 = EnumSet.of(MyEnum.DFTF, MyEnum.DHSF, MyEnum.EDJS);
System.out.println(“Set10 is : ” + set10);
// 11. Using static <E extends Enum<E>> EnumSet<E> of(E e1, E e2, E e3, E e4)
EnumSet<MyEnum> set11 = EnumSet.of(MyEnum.DFTF, MyEnum.DHSF, MyEnum.EDJS, MyEnum.KSOO);
System.out.println(“Set11 is : ” + set11);
// 12. Using static <E extends Enum<E>> EnumSet<E> of(E e1, E e2, E e3, E e4, E e5)
EnumSet<MyEnum> set12 = EnumSet.of(MyEnum.DFTF, MyEnum.FJEK, MyEnum.DHSF, MyEnum.EDJS, MyEnum.KSOO);
System.out.println(“Set12 is : ” + set12);
// 13. Using static <E extends Enum<E>> EnumSet<E> range(E from, E to)
EnumSet<MyEnum> set13 = EnumSet.range(MyEnum.SODJ, MyEnum.DFTF);
System.out.println(“Set13 is : ” + set13);
}
}
Output:
Set1 is : [EDJS, FJEK, SODJ, SEUF, SEF, SERE, DHSF, DFTF, KSOO]Set2 is : [EDJS, FJEK, SODJ, SEUF, SEF, SERE, DHSF, DFTF, KSOO]
Set3 is : []
Set4 is : [FJEK, DFTF, KSOO]
Set5 is : [FJEK, DFTF, KSOO]
Set6 is : []
Set7 is : [DFTF] Set8 is : [SODJ, SEUF, SEF, SERE, DHSF, DFTF, KSOO]
Set9 is : [DHSF, DFTF]
Set10 is : [EDJS, DHSF, DFTF]
Set11 is : [EDJS, DHSF, DFTF, KSOO]
Set12 is : [EDJS, FJEK, DHSF, DFTF, KSOO]
Set13 is : [SODJ, SEUF, SEF, SERE, DHSF, DFTF]