What are ResultSet Constants in JDBC:
We have seen how to connect to the MySQL database and read the data from the table. The data returned after executing the query is of ResultSet type. ResultSet in JDBC is an interface that comes under the java.sql package. In this blog, we will see how to set different types of ResultSet Constants in JDBC.
- A ResultSet object maintains a cursor pointing to its current row of data. Initially, the cursor is positioned before the first row.
- The next() method moves the cursor to the next row, and because it returns false when there are no more rows in the ResultSet object, it can be used in a while loop to iterate through the result set.
- A default ResultSet object is not updatable and has a cursor that moves forward only. Thus, we can iterate through it only once and only from the first row to the last row.
- It is possible to produce ResultSet objects that are scrollable and/or updatable.
- We can also set if we want to hold or close cursors after committing.
We have seen the different types of JDBC Statements. While creating those statements we can also set the Type, Concurrency, and Holdability of the result set. We have different constant values to set these properties as shown below.
ResultSet Type constants:
- ResultSet.TYPE_FORWARD_ONLY: The constant indicating the type for a ResultSet object whose cursor may move only forward. When we are not setting any type, ResultSet has this type by default.
- ResultSet.TYPE_SCROLL_INSENSITIVE: The constant indicating the type for a ResultSet object that is scrollable but generally not sensitive to changes to the data that underlies the ResultSet.
- ResultSet.TYPE_SCROLL_SENSITIVE: The constant indicating the type for a ResultSet object that is scrollable and generally sensitive to changes to the data that underlies the ResultSet
ResultSet Concurrency constants:
- ResultSet.CONCUR_READ_ONLY: The constant indicating the concurrency mode for a ResultSet object that may NOT be updated. When we are not setting any concurrency then this is the default value set.
- ResultSet.CONCUR_UPDATABLE: The constant indicating the concurrency mode for a ResultSet object that may be updated.
ResultSet Holdability constants:
- ResultSet.HOLD_CURSORS_OVER_COMMIT: The constant indicating that open ResultSet objects with this hold ability will remain open when the current transaction is committed.
- ResultSet.CLOSE_CURSORS_AT_COMMIT: The constant indicating that open ResultSet objects with this hold ability will be closed when the current transaction is committed.
Note that sample codes can refer to JDBC Statements blog.
We will see one simple example for type and concurrency.
First, we will not set any type and will try to move the cursor back as shown in the below example. Since no type is set, the default type will be TYPE_FORWARD_ONLY.
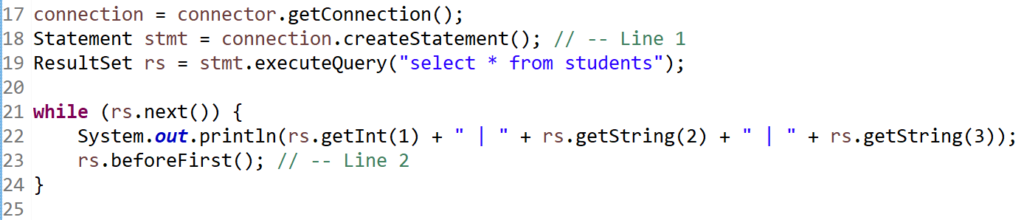
As we can see from line 1 we have created the statement without any result set type value mentioned. Hence when we are calling the rs.beforeFirst() method as seen in line 2. We will get the following exception after reading the first row.
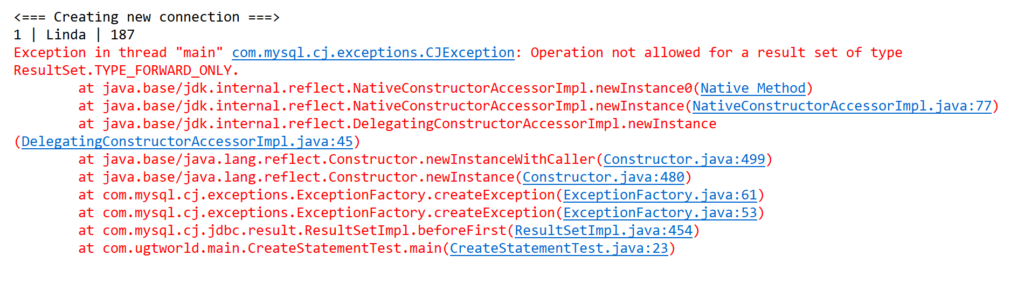
If we set the type and concurrency as shown below, we will not get any exceptions. Since the cursor is moving to the next and previous row continuously we will get the infinite loop printing result of the first record.

The syntax for the Create statement to set type, concurrency, and hold ability:
- Statement createStatement(int resultSetType, int resultSetConcurrency)
- Statement createStatement(int resultSetType, int resultSetConcurrency, int resultSetHoldability)
The syntax for Prepared Statement to set type, concurrency, and hold ability:
- PreparedStatement prepareStatement(String query, int resultSetType, int resultSetConcurrency)
- PreparedStatement prepareStatement(String sql, int resultSetType, int resultSetConcurrency, int resultSetHoldability)
The syntax for Callable Statement to set type, concurrency, and hold ability:
- CallableStatement prepareCall(String query, int resultSetType, int resultSetConcurrency)
- CallableStatement prepareCall(String sql, int resultSetType, int resultSetConcurrency, int resultSetHoldability)