How Abstraction works in java
We have got an overview of Abstraction in the Introduction of Java. In this blog, we will see how Abstraction works in java and examples of Abstraction.
What is Abstraction?
Data Abstractions is the process of hiding the implemented details and showing only essential details to the user. For example, in the ATM machine if the user wants to check a balance or withdraw the money he follows the steps and does the action, but in detail what user is not aware of the internal implementation of the steps.
We can achieve abstraction in 2 ways in java.
- By using Abstract class.
- By using an Interface.
By Using Abstract Class :
When we add an abstract keyword in front of the class name then that class becomes an abstract class. An abstract class can have both abstract and non-abstract methods.
Example:
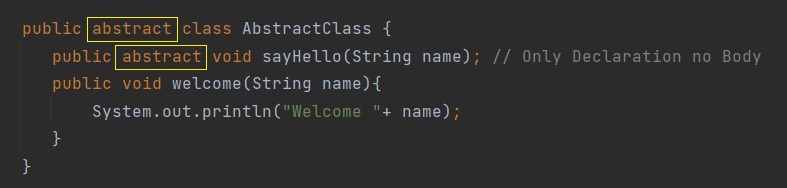
Abstract Method: This method also has an abstract keyword added. Abstract methods only have declarations. This method doesn’t have a body, this needs to be implemented by the subclass/child class of an abstract class.
Important Points for Abstract Class:
- We should add an abstract keyword for the abstract class.
- An abstract class can have both abstract and non-abstract methods.
- If any class is having at least one abstract method then we must declare that class as an abstract class.
- An abstract class can not be initiated. We will get the compile-time error as follows:
“Error: class is abstract; cannot be instantiated” - An abstract class can have constructors and static methods.
- Child class must implement all abstract methods of its parent abstract class. Or else it should be declared as an abstract class and its child can implement the abstract methods of the first parent class.
Example: If abstract class A has 2 abstract methods m1 and m2, and class B extends Class A, then B should have implementation for both m1 and m2. Or B can be declared as abstract and its Child (if Class C is extending B) must have implementation for m1 and m2.
We will get the following compile-time error if class B is not abstract.
“Error: Class is not abstract and does not override abstract method”.
Why should we use abstract class and not interface?
- An abstract class can have non-abstract methods as well (though from java 1.8 onwards we can also add default and static methods in an interface).
- Once the interface is used, we can not modify anything whereas in abstract class we can modify the class without breaking the existing behavior.
- The abstract class allows implementation partly in the child class. Whereas interface does not allow it.
- Hence if we want to create multiple versions then we should go for an abstract class instead of an interface.