ArrayList is a mostly used collection class in java. This is used to store different lists of objects
We have seen List in Java. Now we will see how we can store elements in ArrayList in java and how to use different methods for array lists.
ArrayList
- java.util.ArrayList is a mostly used collection class in java. This is used to store different lists of objects.
- Since in java array we need to define size at the time of declaration itself as shown.
String[] strArray = new String[5];
In the above, we can not increase the size of an array later since the maximum size of an array is 5.
- To overcome this issue we can use ArrayList to store the data. Array list starts with default size – 10. Array List grows automatically when more data is added to it.
- We can also provide initial capacity to an array list while creating an object.
ArrayList has Three ways to create an object.
- ArrayList<String> list = new ArrayList<String>();
This will create an empty list with initial default capacity.
- ArrayList<String> list = new ArrayList<String>(20);
This will create an empty list with a capacity of 20. Here 20 is the initial capacity. When we know that array is required to store huge data so we can initially create the array with that capacity this will save the time required to grow and array and reallocate the elements. We can not pass negative numbers as initial capacity else IllegalArgumentException will be thrown.
- ArrayList<String> list = new ArrayList<String>(oldList);
This will create an object by taking another list as a parameter and add into the new array list in the sequence it’s returned by an iterator. This will throw NullPointerException if oldList is null.
Let’s see the example below for the using above 3 constructors.
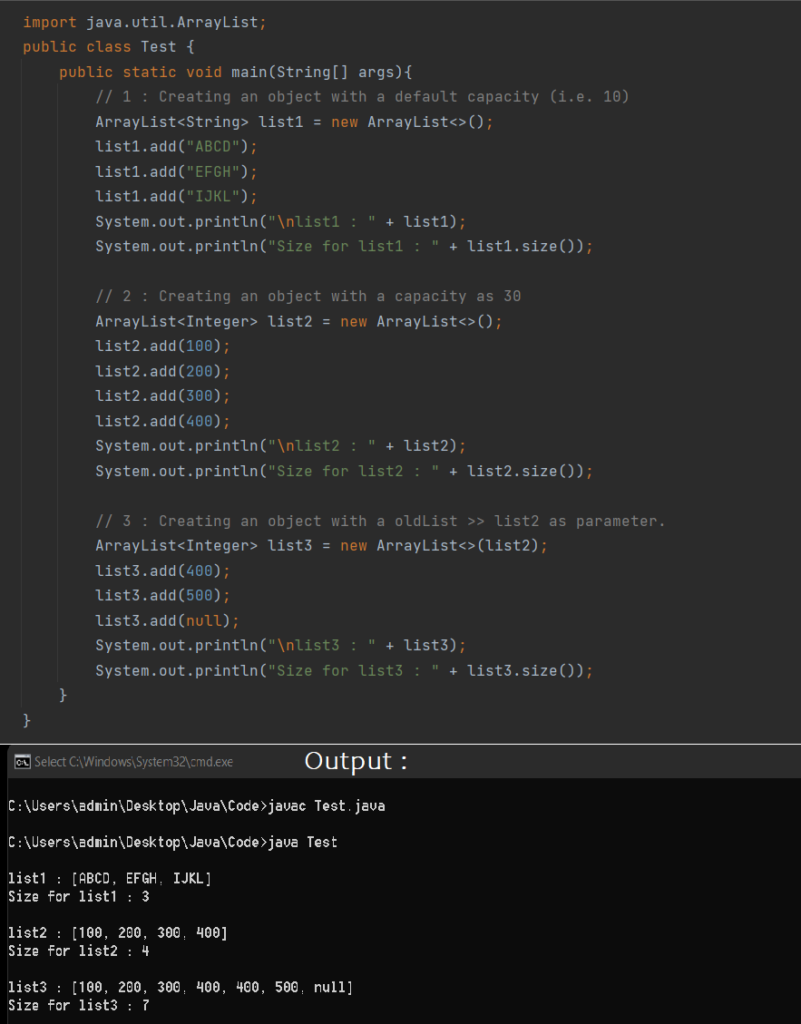
Let’s see different ArrayList methods that are commonly used.
- add(E e),
- add(int index, E e),
- addAll(Collection <? extends E> c),
- addAll(int index, Collection <? extends E> c)
These methods are used for adding elements or another list into another list. With index position given that element/list will be added at that index position else, it will be added at the end (appended) of the list. An example for add is as below.
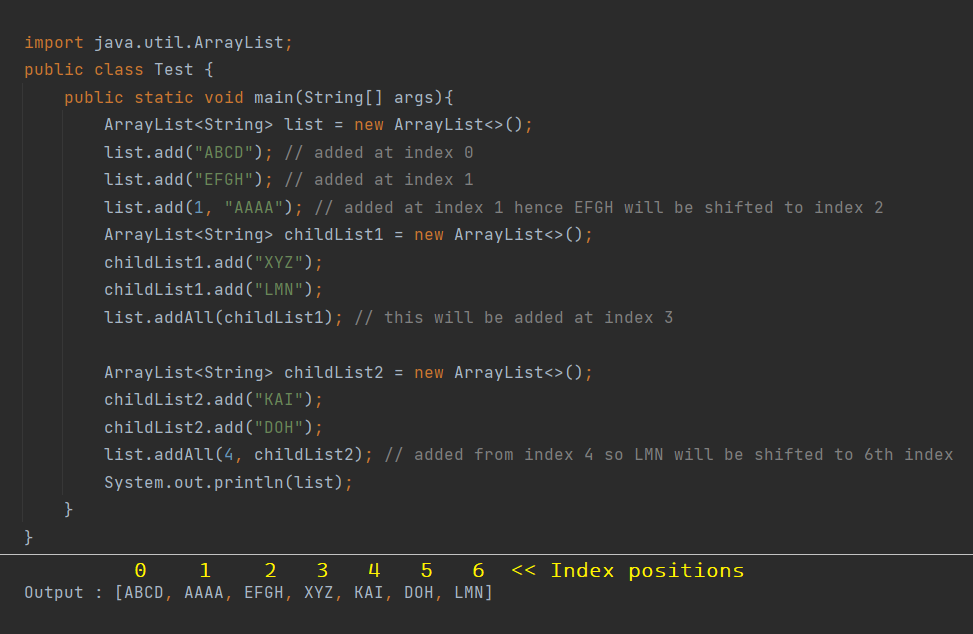
- contains(E e) : To check if element is present inside the list.
- isEmpty(): To check if list is empty.
- indexOf(E e): To check the index position of an element (first occurrence).
- lastIndexOf(E e): This will return index position of last occurrence of element.
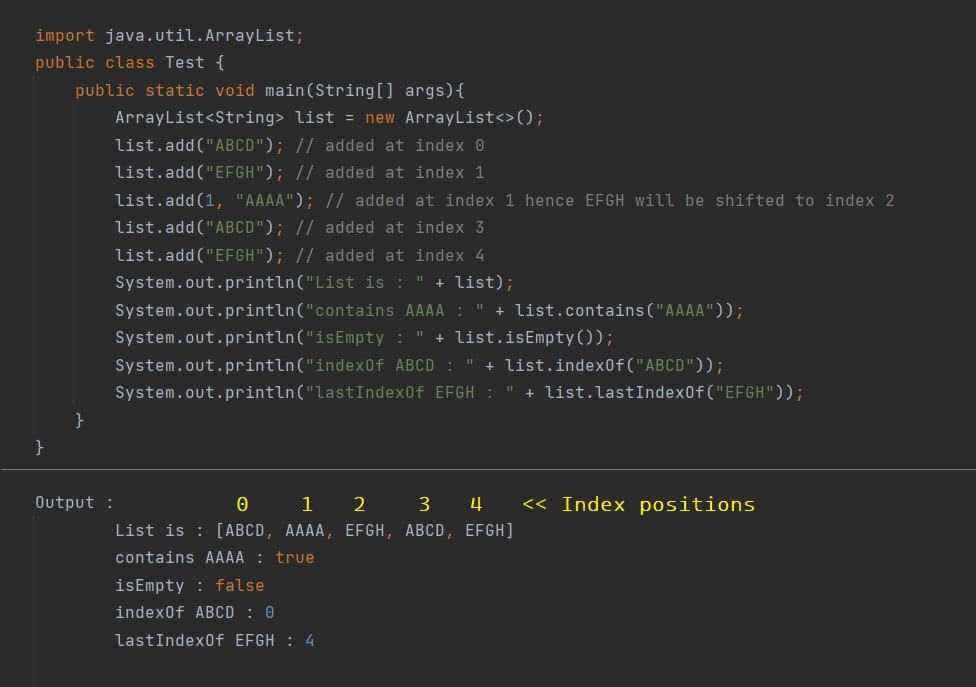
- forEach(Consumer<? super E> action) : iterate over each element in the list.
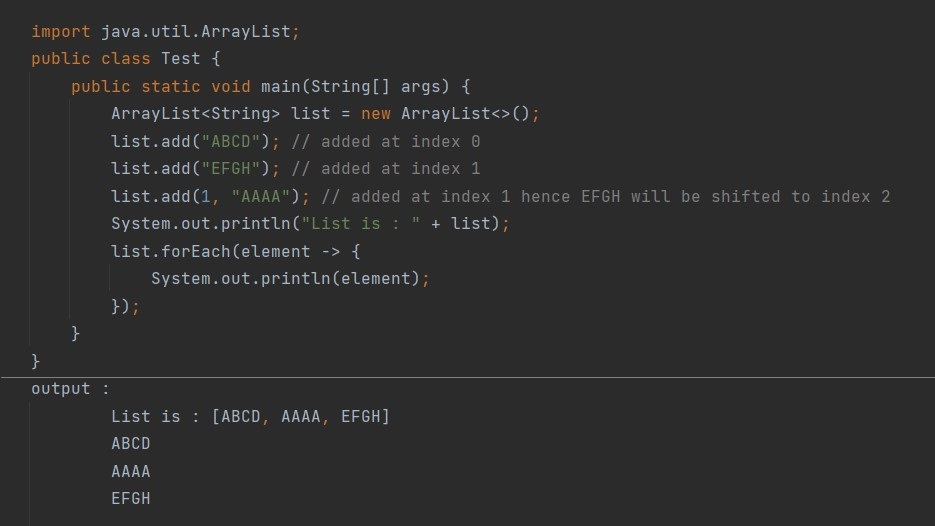
- iterator(): returns the iterator for the elements in the list, it returns a fail-fast iterator (i.e operations are performed on the original list). For the above list instead of forEach we can also print elements using java.util.Iterator
- listIterator(): This returns the list iterator which is also a fail-fast iterator. This is the same as the Iterator shown above. We are using java.util.ListIterator
- listIterator(int index): This returns the list iterator starting from the specified index. In the below example elements will be printed starting from index 1.
- clear(): removes all the elements from the list as shown in the below example.
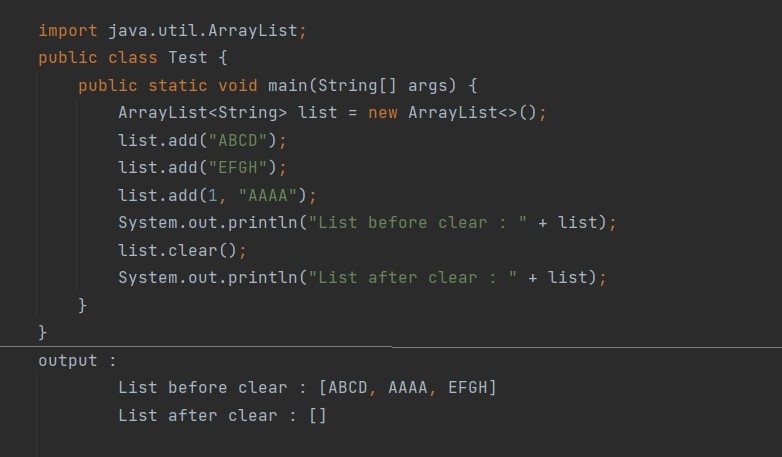
- remove(int index): removes the element from specified index position.
- remove(Element e): removes an element from the list if it’s present. If that element is not present then the list will remain as it is.
- removeAll(Collection c): Removes all the elements given in the collection from the list if present.
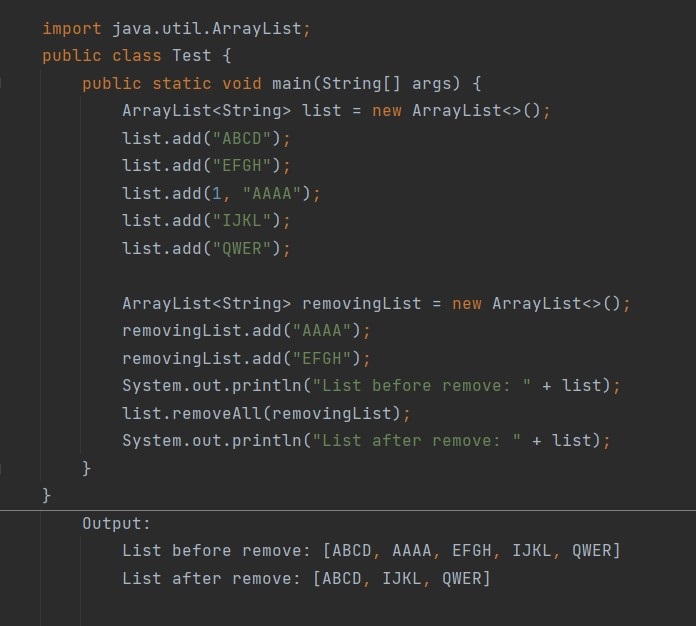
- removeIf(Predicate<? super E> filter): Removes all the elements from the list which satisfy the given condition.
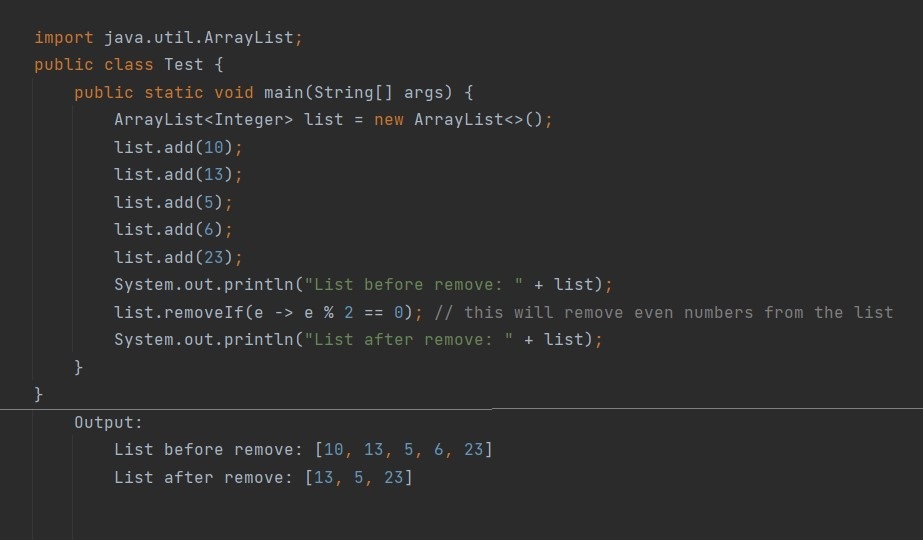
- retainAll(Collection<E> c): Retains only those elements which are present in the provided collection and deletes the remaining.
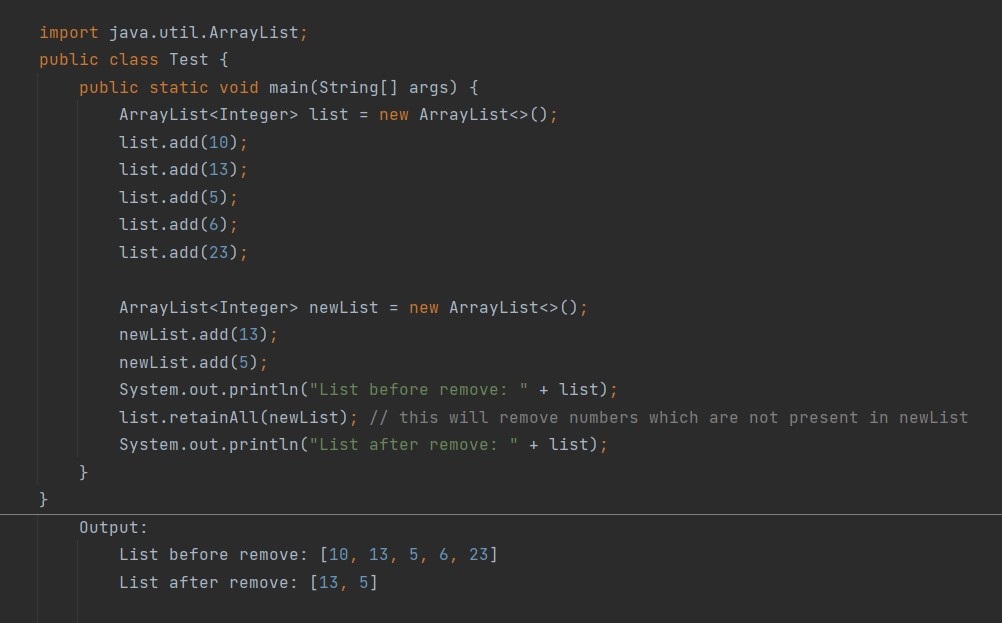
- replaceAll(UnaryOperator<E> operator): Replaces each element of this list with the result of unary operations.
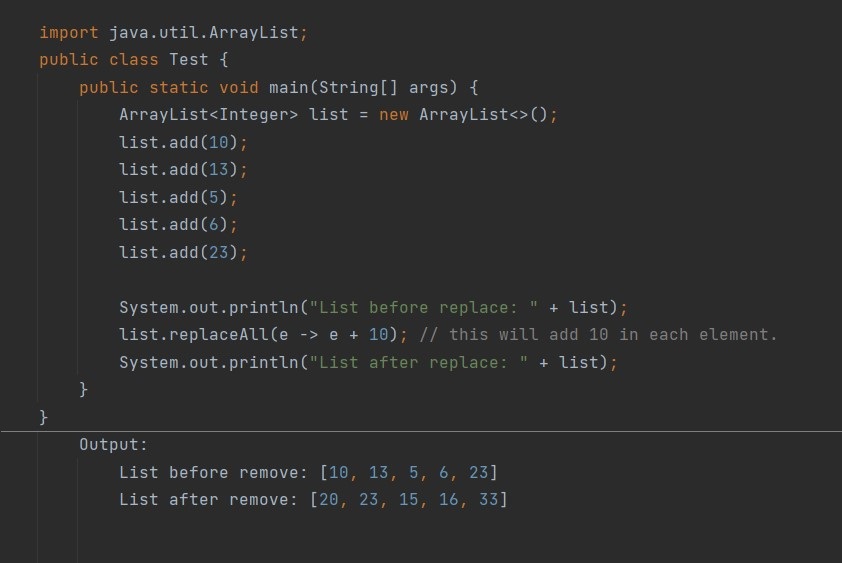
- get(int index): To get the element from a particular index position.
- set(int index, E e): to replace the element at a particular index position.
- subList(int fromIndex, int toIndex): To get the sublist from an original list with from index(inclusive) and to index(exclusive) specified.
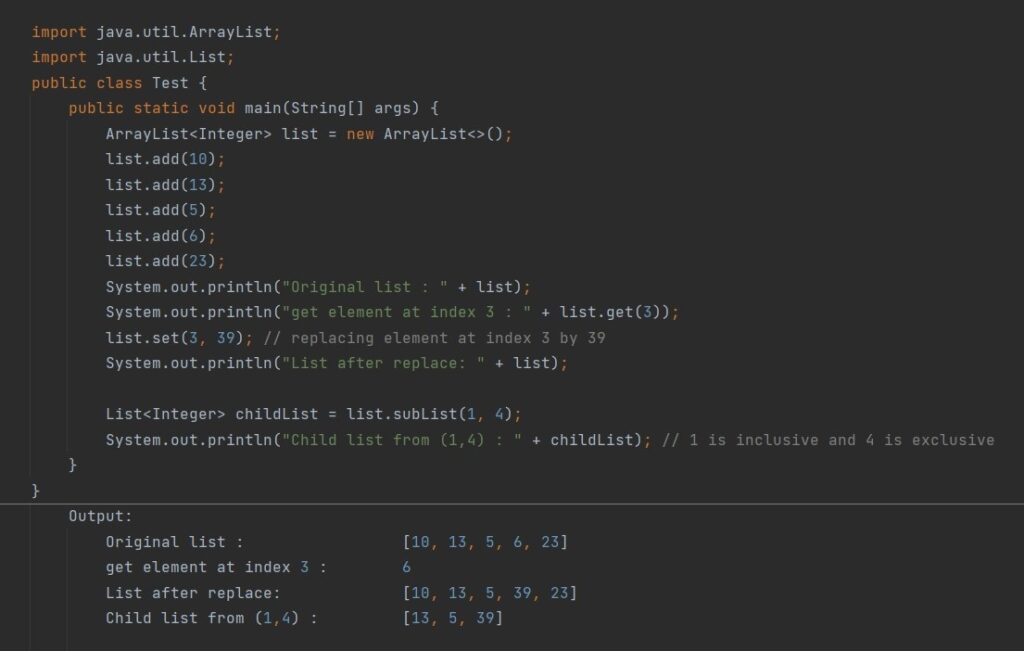
- spliterator(): To create late-binding and fail-fast Spliterator. Using forEachRemaining method of Spliterator we can iterate over the element o the list.
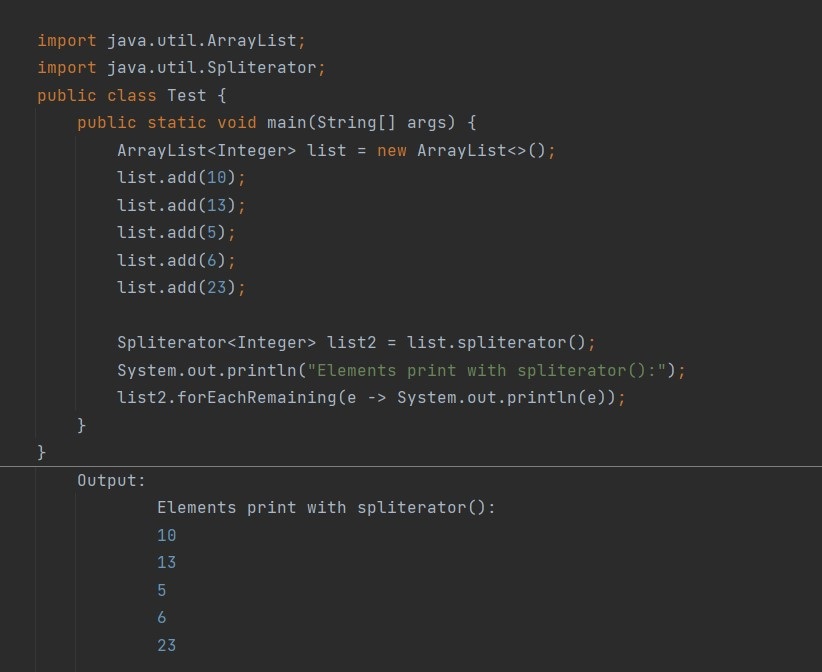
- ensureCapacity(int minCapacity): To increase the capacity of ArrayList instance with the minCapacity provided.
- size(): This will return the size of the list (no. of elements present in the list).
- trimToSize(): This will trim the capacity of the list to its actual size.
- sort(Comparator<? super E> c): This will sort the
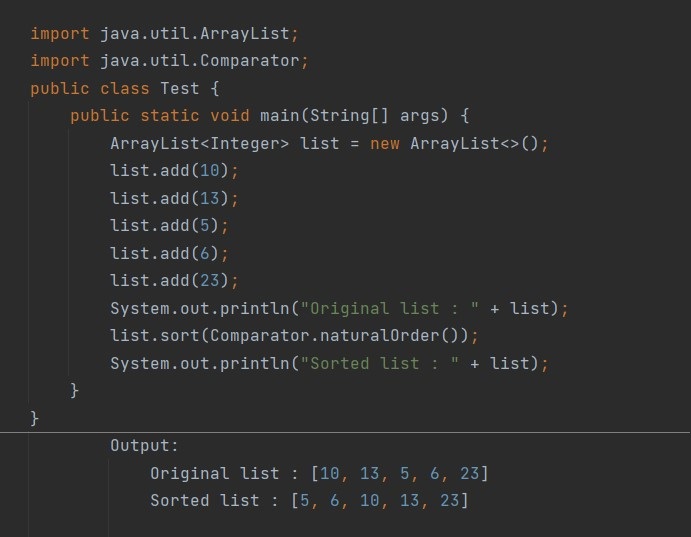
- toArray(): This will return the new array object with all the elements from first to last in the sequence.
- toArray(T[] a): This will return the new array object where the runtime type of the returned array is that of the specified array.
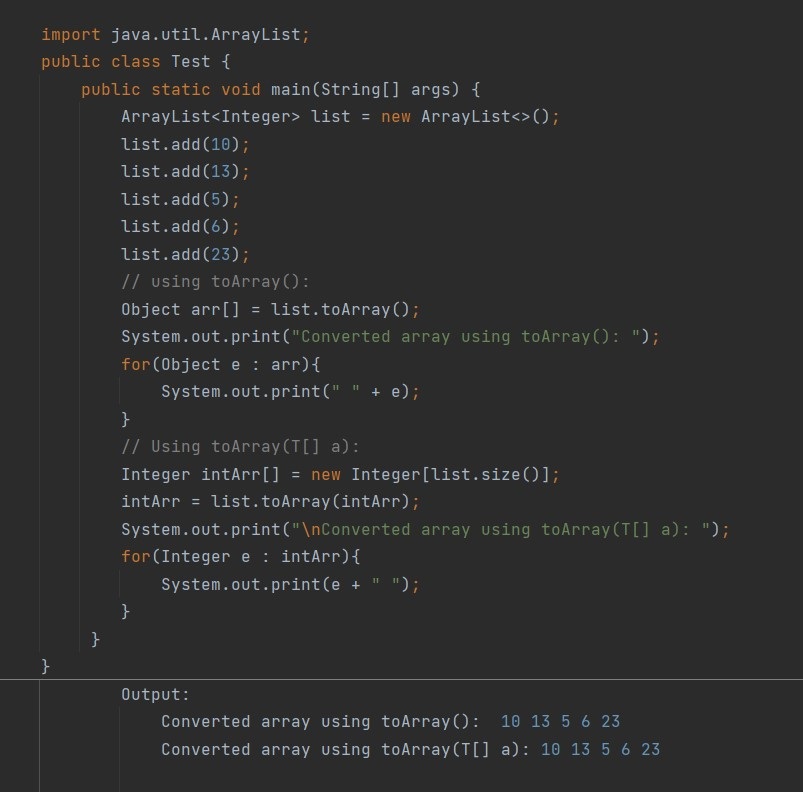