What is swagger in SpringBoot?
Swagger in SpringBoot allows you to describe the structure of your APIs so that machines can read them. The ability of APIs to define their own structure is the root of all the awesomeness in Swagger.
Swagger allows us to build interactive API documentation.
We can also automatically generate client libraries for our API in many languages and explore other possibilities like automated testing. Swagger does this by asking your API to return a YAML or JSON that contains a detailed description. This file is essentially a resource listing of your API which adheres to OpenAPI Specification. The specification needs to include information like:
- What are all the operations that API supports?
- What are API’s parameters and what does it return?
- Does API need some authorization?
- We can even add terms, contact information, and licenses to use the API.
We can write a Swagger spec for API manually or have it generated automatically from annotations in the source code.
We can add a swagger to our spring boot application like below.
We need to add spring doc open API started dependency for webmvc-ui as shown in below.
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-starter-webmvc-ui</artifactId>
<version>2.0.2</version>
</dependency>
Note: For spring boot 3.x make sure to use 2.x.x version of openapi.
Once the above dependency is added to our pom.xml, we can re-run our application to check the swagger-ui.
The link for swagger-ui will be as follows. We can refer the full code from here…
http://localhost:<port>/swagger-ui/index.html
We have already created multiple APIs in our spring boot project in the previous blog ‘Request mappings in spring boot’
We can see the following swagger UI like below.
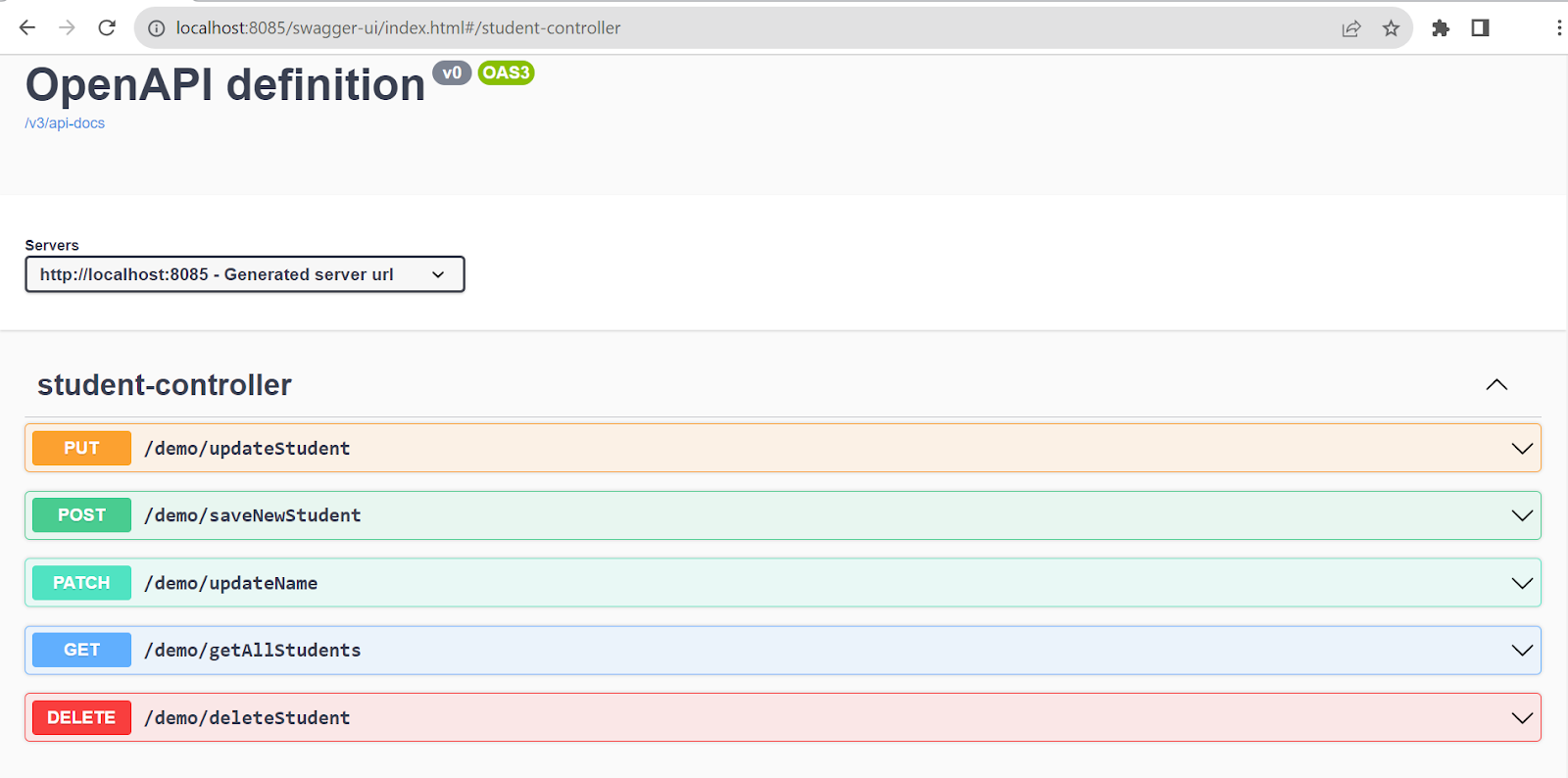
All the APIs present in our project will be listed on the Swagger UI, We can expand the API and try it out, and execute the API as shown below.
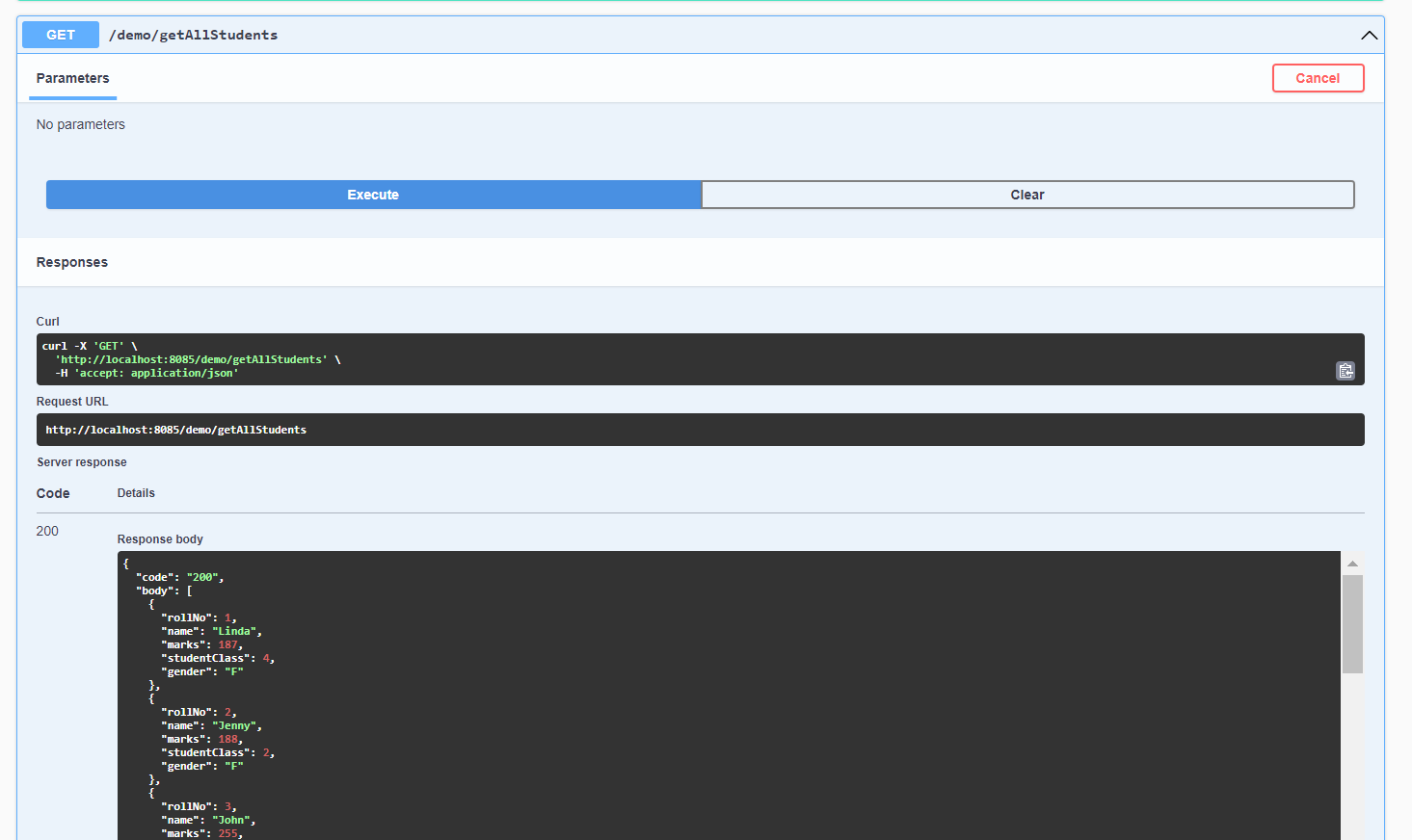
If we need to send any request we can send the request body or request parameter like below.
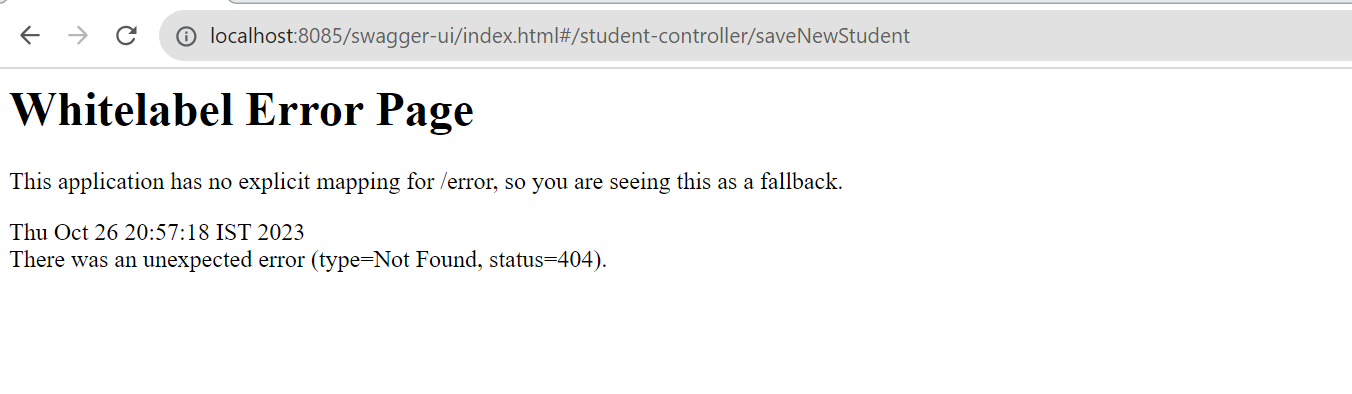
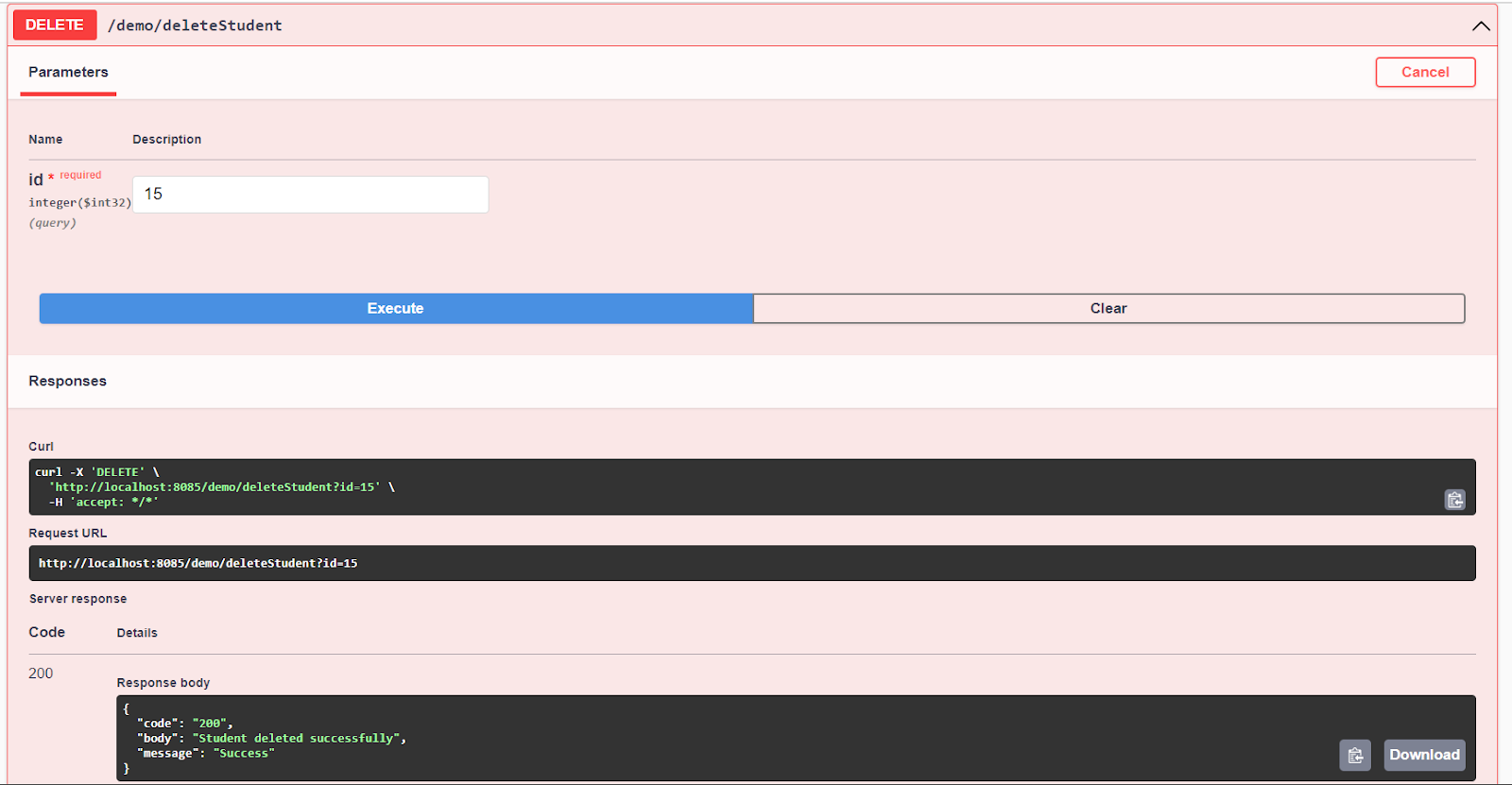
In SprinBoot, once an open API dependency is added for the swagger, the default configuration is internally done by SprinBoot. Properties for swagger UI and api-docs by default will be marked as enabled=true.
If we need to disable the same, we can include the following properties in the application.properties file and mark them as false.
We can enable them whenever required by simply making them true.
springdoc.swagger-ui.enabled=false
springdoc.api-docs.enabled=false
Once these properties are marked as false we won’t be able to load the swagger UI and we will get the following page instead.
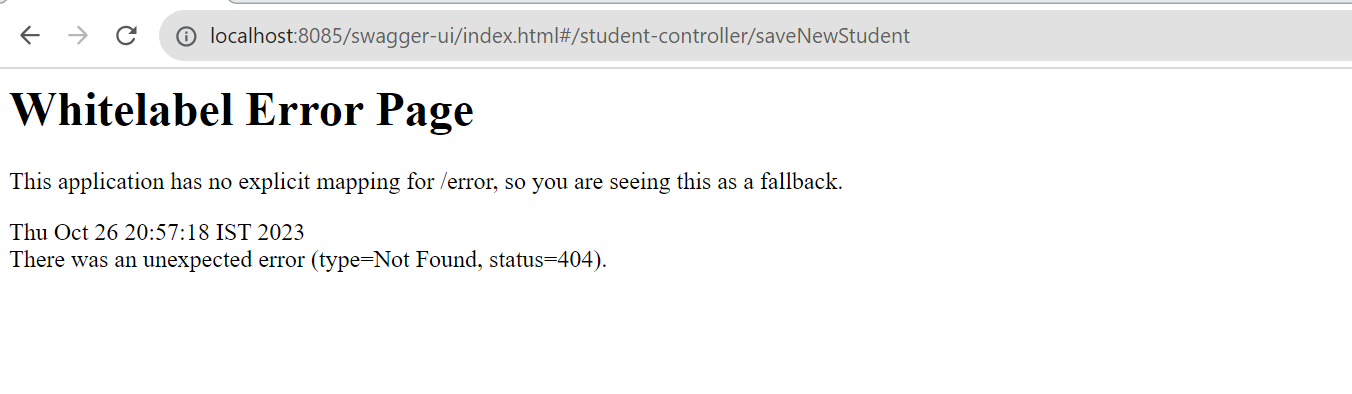