The most common class used in java is the String class
In this blog, we will see the features of the String class. Java String class is defined in java.lang package.
Java String class
The String is not a primitive type, when we create any string it creates the object of type String. The string is immutable which means we can not change the value of the String object. If we assign a different value to the String variable then the new object will get created.
To create mutable string objects java provides StringBuffer and StringBuilder. StringBuilder is faster than StringBuffer but if thread safety is need then StringBuffer is preferred.
Let’s see how to create a string object:
1. Using String Literal
This is the most common way used to create string objects.
String str = “ugt”;
If we create and initialize the string using the string literal method, JVM checks if any value is present in a string pool. If any value is present then that memory location reference. If no value is found in the string pool then a new memory location is created and value is stored in that location and this new location reference is returned.
If we try to create another string with the same value then both the objects will refer to the same memory location instead of creating a new memory location same reference is returned to the new object.
Let’s check the example for creating multiple string objects and how memory is allocated in the string pool.
If we check if the str and str2 are equals then it will print true since they are referring to the same string pool memory location (address is same as shown in the below example).
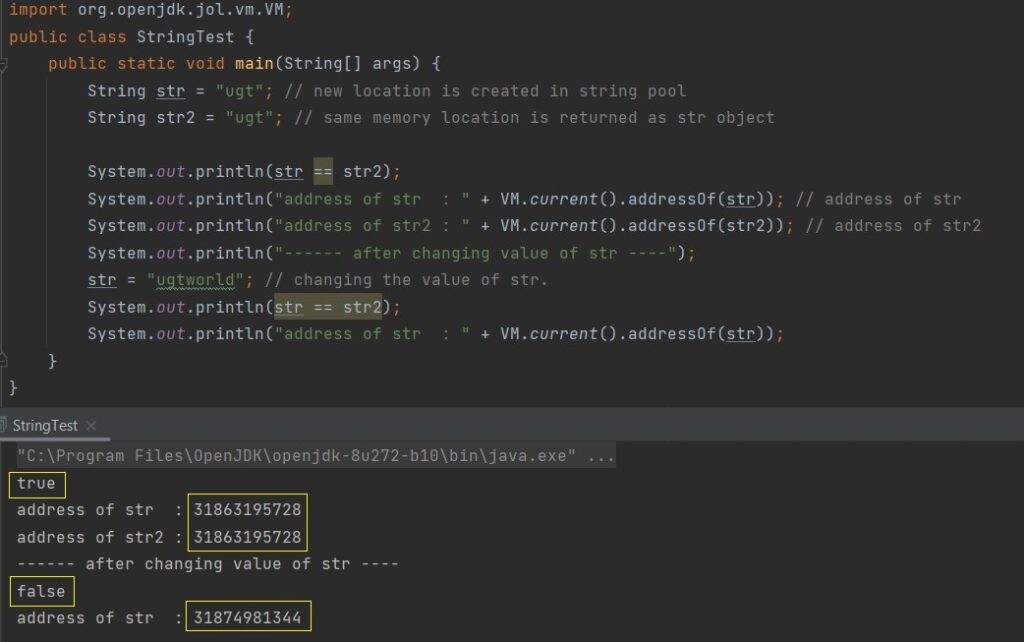
In the above example if we change the value of str then the new object will be created and the new location will be returned hence if we check the two strings are equals then it will print false.
Below is the diagram of how memory is allocated in the string pool.
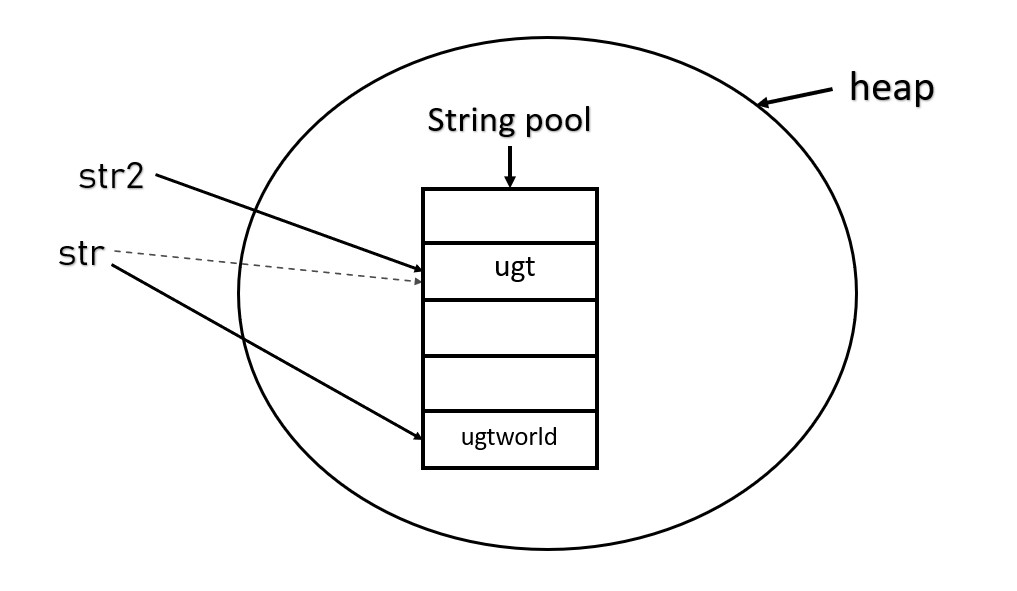
In the above diagram, str is first referring to the value ugt, same reference is given to str2. Once we change the value of str then the new location is referred by str as shown.
Since the string is immutable this feature of string pooling helps to save the memory and hence it is more secure since value can not be changed and is thread-safe.
2. Using new keyword
We can also create the string object by using the new keyword. Each time we create the new string object, the new object will get created in java heap memory even if the value is the same for them. Hence memory location (address) for both str and str2 will be different.
Example to create string object using the new keyword :
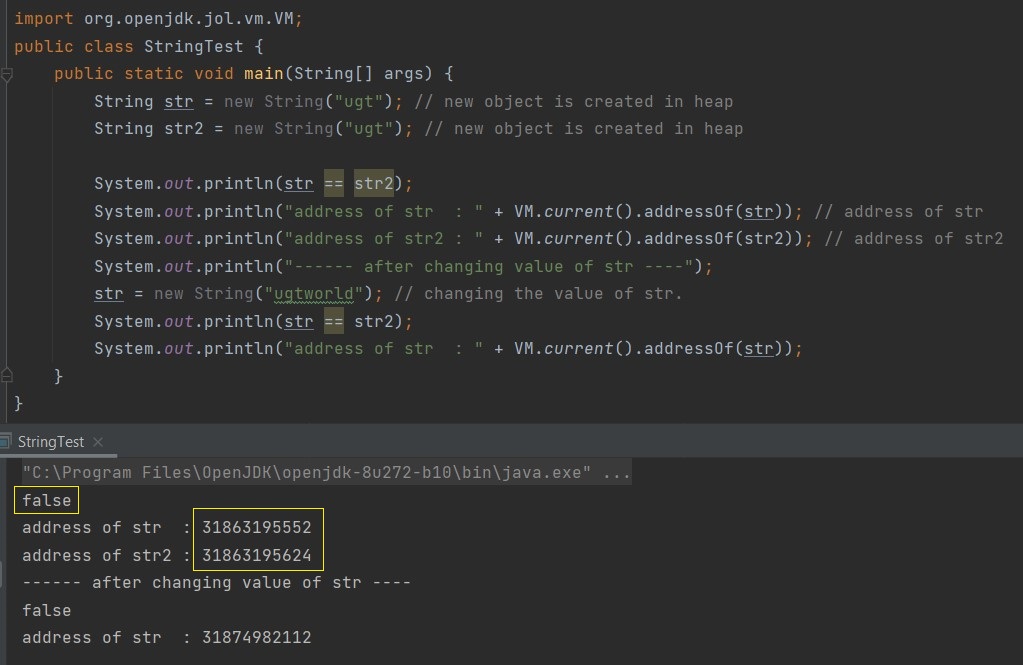
We can see that in the above example address printed for both the str and str2 is different even if the value is the same, also if we check if the strings are equal it’s returning false.
In the following diagram, we can see how the objects are created in heap.
Note that if we just want to check the value of the two strings irrespective of the address or if two objects are the same or different it’s recommended to use the equals method than ‘==’. This equals method will compare the value of two strings and return true if the value matches.
So in the previous example if we replace == by equals method we will get true.
String str = new String("ugt"); // new object is created in heap
String str2 = new String("ugt"); // new object is created in heap
System.out.println(str.equals(str2));
Java provides different inbuild methods inside the String class to perform different operations on the string. We will see the example for each string method later.
Note that to print the address of string new dependency/jar needs to be added and we should import it in our example :
import org.openjdk.jol.vm.VM;
Dependency for above jar is :
<dependency>
<groupId>org.openjdk.jol</groupId>
<artifactId>jol-core</artifactId>
<version>0.10</version>
</dependency>
Or we can directly download the jar and import it from the following site
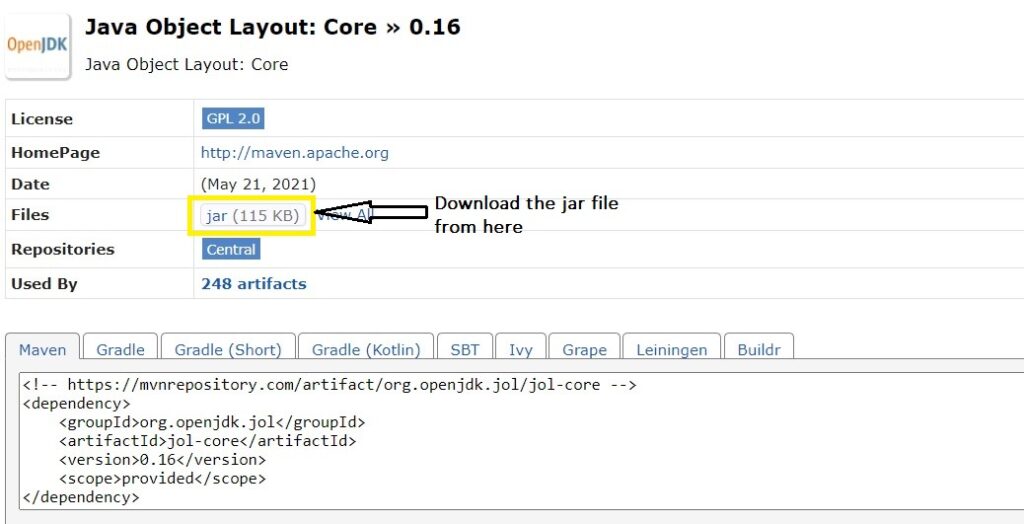