If we want to store a list of elements in java, Java has a List interface in java.
List in java allows us to maintain the ordered collection of objects. We can insert, delete, update the elements in the list based on index position.
We can store duplicate elements inside the list. List interface is a child of Collection interface and comes under java.util package.
Implementation classes for the list interface are ArrayList, LinkedList, Vector, Stack as shown in the below diagram
List interface provides different methods:
Method | Description |
boolean add(E e) | Appends the element at the end. |
void add(int index, E element) | Inserts element at the specified index position. |
boolean addAll(Collection<? extends E> c) | Appends all the elements in the specified collection to the end of the list. |
void clear() | Removes all of the elements from this list. |
boolean contains(Object o) | Checks if a list contains the specified element and returns true if present. |
boolean containsAll(Collection<?> c) | Returns true if the list contains all of the elements of the specified collection. |
boolean equals() | Compares the specified object with the list for equality. |
E get(int index) | Returns the element at the specified position. |
int hashCode() | Returns the hashcode value. |
int indexOf(Object o) | Returns the index of the first occurrence of the specified element in the list, or -1 if the list does not contain the element. |
boolean isEmpty() | Checks if list is empty. |
Iterator<E> iterator() | Returns an iterator over the elements in the list in proper sequence. |
ListIterator<E> listIterator() | Returns a list iterator over the elements in the list (in proper sequence). |
ListIterator<E> listIterator(int index) | Returns a list iterator over the elements in the list (in proper sequence), starting at the specified position in the list. |
int lastIndexOf(Object o) | Returns the index of the last occurrence of the specified element in the list, or -1 if the list does not contain the element. |
E remove(int index) | Removes the element at the specified position in the list (optional operation). |
boolean remove(Object o) | Removes the first occurrence of the specified element from the list, if it is present. |
boolean removeAll(Collection<?> c) | Removes from the list all of its elements that are contained in the specified collection. |
default void replaceAll(UnaryOperator<E> operator) | Replaces each element of the list with the result of applying the operator to that element. |
boolean retainAll(Collection<?> c) | Retains only the elements in the list that are contained in the specified collection. |
E set(int index, E element) | Replaces the element at the specified position in the list with the specified element. |
int size() | Returns the number of elements in the list. |
default void sort(Comparator<? super E> c) | Sorts the list according to the order induced by the specified Comparator. |
default Spliterator<E> spliterator() | Creates a Spliterator over the elements in the list. |
List<E> subList(int fromIndex, int toIndex) | Returns a view of the portion of the list between the specified fromIndex, inclusive, and toIndex, exclusive. |
Object[] toArray() | Returns an array containing all of the elements in the list in the proper sequence from the first to the last element. |
<T> T[] toArray(T[] a) | Returns an array containing all of the elements in the list in the proper sequence from the first to the last element. The runtime type of the returned array is that of the specified array. |
Let’s see the example for list creation insert and iterate over the list. In the below example we have created an ArrayList object of String type elements. Index position in the list starts from 0. In the below list, the size is 3 hence we can get the elements at index 0, 1, and 2. If we try to get the element at index 3 then we will get a Runtime exception saying “java.lang.IndexOutOfBoundsException: Index: 3, Size: 3”.
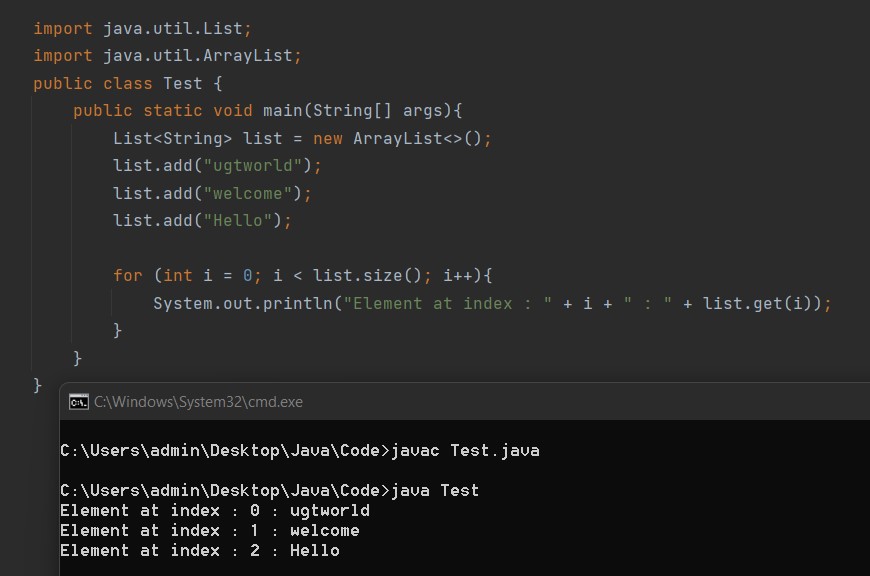