What are the new features of java-11?
On September 2018, Oracle released a new version of Java as Java-11. Following are the new features of java-11.
Java 11 is Long term support (LTS) after java 8. Oracle stopped supporting Java 8 in January 2019. As a consequence, a lot of us will upgrade to Java 11.
We will explore new features, removed features, and performance enhancements introduced in Java 11.
- New String Methods: New methods are added to the String class.
- isBlank(): This will check if the string is blank. It will return true if the string is blank.
- strip(): This will Return a string whose value is this string, with all leading and trailing white space removed.
- stripLeading(): Returns a string whose value is this string, with all leading white space removed.
- stripTrailing(): Returns a string whose value is this string, with all trailing white space removed.
- lines(): This will return a stream of lines extracted from this string, separated by line terminators.
- repeat(): Returns a string whose value is the concatenation of this string repeated count times. If this string is empty or the count is zero then the empty string is returned.
import java.util.stream.Stream; public class Test { public static void main(String[] args) { String str1 = “”, str2 = ” “, str3 = ” ab d “; // 1. isBlank() System.out.println(“—- 1. isBlank() —-“); System.out.println(“str1 is blank: ” + str1.isBlank()); System.out.println(“str2 is blank: ” + str2.isBlank()); System.out.println(“str3 is blank: ” + str3.isBlank()); // 2. strip() System.out.println(“—- 2. strip() —-“); System.out.println(“str1.strip():-” + str1.strip()+”-:”); // blank System.out.println(“str2.strip():-” + str2.strip()+”-:”); // blank System.out.println(“str3.strip():-” + str3.strip()+”-:”); // ab d // 3. stripLeading System.out.println(“—- 3. stripLeading() —-“); System.out.println(“str1.strip():-” + str1.stripLeading()+”-:”); System.out.println(“str2.strip():-” + str2.stripLeading()+”-:”); System.out.println(“str3.strip():-” + str3.stripLeading()+”-:”); // 4. stripTrailing System.out.println(“—- 4. stripTrailing() —-“); System.out.println(“str1.strip():-” + str1.stripTrailing()+”-:”); System.out.println(“str2.strip():-” + str2.stripTrailing()+”-:”); System.out.println(“str3.strip():-” + str3.stripTrailing()+”-:”); // 5. lines() System.out.println(“—- 5. lines() —-“); String str = “ugtworld \r test \n this is next line”; Stream<String> lines = str.lines(); lines.forEach(a -> System.out.println(a.strip())); // 6. repeat() System.out.println(“—- 6. repeat() —-“); String val = “ABCD”; System.out.println(val.repeat(2)); } }
Output:
—- 1. isBlank() —- str1 is blank: true str2 is blank: true str3 is blank: false —- 2. strip() —- str1.strip():–: str2.strip():–: str3.strip():-ab d-: —- 3. stripLeading() —- str1.strip():–: str2.strip():–: str3.strip():-ab d -: —- 4. stripTrailing() —- str1.strip():–: str2.strip():–: str3.strip():- ab d-: —- 5. lines() —- ugtworld test this is next line —- 6. repeat() —- ABCDABCD- New File Methods:
- new readString() and writeString(), and isSameFile() methods are added to read, write and check if the file path is same.
import java.io.File; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; public class Test { public static void main(String[] args) throws IOException { String path = “”; File file1 = new File(“testfile.txt”); // testfile.txt will be created in current dir. Path filePath = Paths.get(“”, “”, “testfile.txt”); // 1. writeString() – to write Files.writeString(filePath, “This is ugtworld.com”); // 2. readString() – to read String fileContent = Files.readString(filePath); System.out.println(fileContent); // 3. isSameFile() – to check whether two paths locate the same file or not. System.out.println(Files.isSameFile(filePath, Path.of(“testfile.txt”))); } }
Output:
This is ugtworld.com true- Collection to an Array:
- By using toArray() we can create an array from a collection object.
import java.util.ArrayList; import java.util.List; public class Test { public static void main(String[] args) { List<String> list = new ArrayList<>(); list.add(“DKS”); list.add(“KJM”); list.add(“KJD”); list.add(“BBH”); list.add(“PCY”); String[] strArr = list.toArray(String[]::new); System.out.println(strArr[3]+ “, ” + strArr[4]); } }
Output:
op BBH, PCY- var keyword for Lambda:
- we can now use the var keyword inside the lambda function.
import javax.annotation.Nonnull; import java.util.ArrayList; import java.util.List; import java.util.stream.Collectors; public class Test { public static void main(String[] args) { List<String> list = new ArrayList<>(); list.add(“DKS”); list.add(“KJM”); list.add(“KJD”); list.add(“BBH”); list.add(“PCY”); String resultString = list.stream() .map((@Nonnull var x) -> x.toLowerCase()) .collect(Collectors.joining(“, “)); System.out.println(resultString); } }
Output:
dks, kjm, kjd, bbh, pcy- not() method for Predicate:
- As shown below now we can perform the not operation on the predicate.
import javax.annotation.Nonnull; import java.util.ArrayList; import java.util.Arrays; import java.util.List; import java.util.function.Predicate; import java.util.stream.Collectors; public class Test { public static void main(String[] args) { List<Integer> list = Arrays.asList(23,54,21,34,56,43,26,98,57); List<Integer> evenList = list.stream() .filter(a -> a % 2 == 0) .collect(Collectors.toList()); List<Integer> oddList = list.stream() .filter(Predicate.not(a -> a % 2 == 0)) .collect(Collectors.toList()); System.out.println(“evenList: ” + evenList); System.out.println(“oddList: ” + oddList); } }
Output:
evenList: [54, 34, 56, 26, 98] oddList: [23, 21, 43, 57]- Running Java Files:
- Previously we need to compile a java file with the ‘javac’ command and then run the file via the command line.
- From java 11 we can directly compile and run the file at same time as shown in below image.
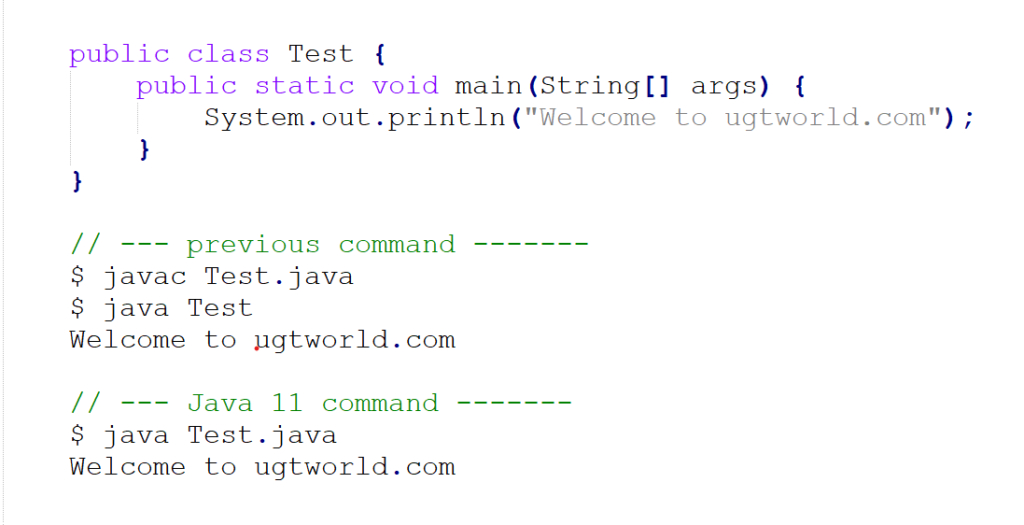
- Pattern Recognizing Methods:
- asMatchPredicate(): This method is similar to Java 8 method asPredicate(). Introduced in JDK 11, this method will Create a predicate that tests if this pattern matches a given input string.
import java.util.function.Predicate; import java.util.regex.Pattern; public class Test { public static void main(String[] args) { String str = “ugtworld”; Predicate t = Pattern.compile(str) .asMatchPredicate(); System.out.println(t.test(“ugtworld”)); System.out.println(t.test(“ugt”)); } }
Output:
true false- Epsilon(No-Op) Garbage Collector:
- A new garbage collector called Epsilon is available for use in Java 11.
- It’s called a No-Op (no operations) because it allocates memory but does not actually collect any garbage.
- Hence Epsilon is applicable for simulating out of memory errors.
- This can be useful for Performance testing, memory pressure testing, VM interface testing, and extremely short-lived jobs.
- TimeUnit Conversion
- This is used to convert the given time to a unit like DAY, MONTH, YEAR, and for time.
import java.time.Duration; import java.util.concurrent.TimeUnit; public class Test { public static void main(String[] args) { System.out.println(“Days: ” + TimeUnit.DAYS.convert(Duration.ofHours(48))); System.out.println(“Minutes: ” + TimeUnit.MINUTES.convert(Duration.ofHours(48))); System.out.println(“Seconds: ” + TimeUnit.SECONDS.convert(Duration.ofHours(48))); } }
Output:
Days: 2 Minutes: 2880 Seconds: 172800Removed Features and Options:
- appletviewer Launcher
- com.sun.awt.AWTUtilities Class
- Java Deployment Technologies
- JavaFX from the Oracle JDK
- JEP 320 Remove the Java EE and CORBA Modules
- JMC from the Oracle JDK
- JVM-MANAGEMENT-MIB.mib
- Lucida Fonts from Oracle JDK
- Oracle JDK’s javax.imageio JPEG Plugin No Longer Supports Images with alpha
- SNMP Agent
- sun.locale.formatasdefault Property
- sun.misc.Unsafe.defineClass
- sun.nio.ch.disableSystemWideOverlappingFileLockCheck Property
- Thread.destroy() and Thread.stop(Throwable) Methods
Deprecated Features and Options
- Deprecate -XX+AggressiveOpts
- Deprecate Stream-Based GSSContext Methods
- JEP 335 Deprecate the Nashorn JavaScript Engine
- JEP 336 Deprecate the Pack200 Tools and API
- Obsolete Support for Commercial Features
- ThreadPoolExecutor Should Not Specify a Dependency on Finalization