Some example scenarios for method overloading:
Example-1:
- In the below example we have two overridden methods first one is for both inputs as integer and second one is for the first input as an integer and the second one is float.
- If we are calling by passing int, int then the first method will get called at compile time compiler will check for a method that matches with the exact parameters, if no exact match found for input object then the compiler will check for any parent object input type (in case of primitive data types it will check for upper casted type means if for int method is not found then it will check for long > float > double).
- So from the below example if we remove the first method then by calling test.getSum(3, 6) will also call the second method.
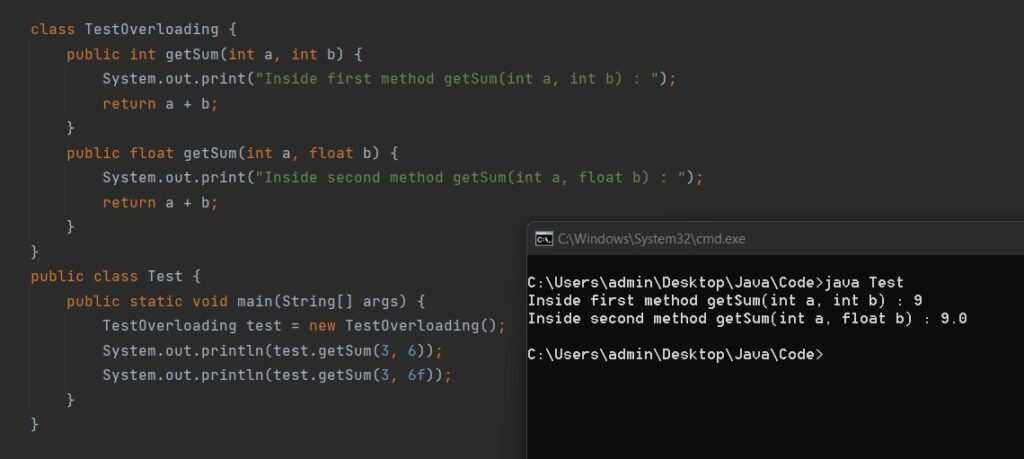
Example 2:
What if we have 2 different methods for (int, float) and for (float, int)?
- If we have both getSum(int a, float b) and getSum(float a, int b) this is also a method overloading and if we are calling these methods by passing (1, 5.3f) and (3.4f, 5), first and the second method will get called respectively without any errors.
- But what if we pass both integer values? Then at the compile-time compile will not be able to decide which method to call since both methods are compatible for input (int, int). Hence this time we will get a compile-time error as: reference to getSum is ambiguous.
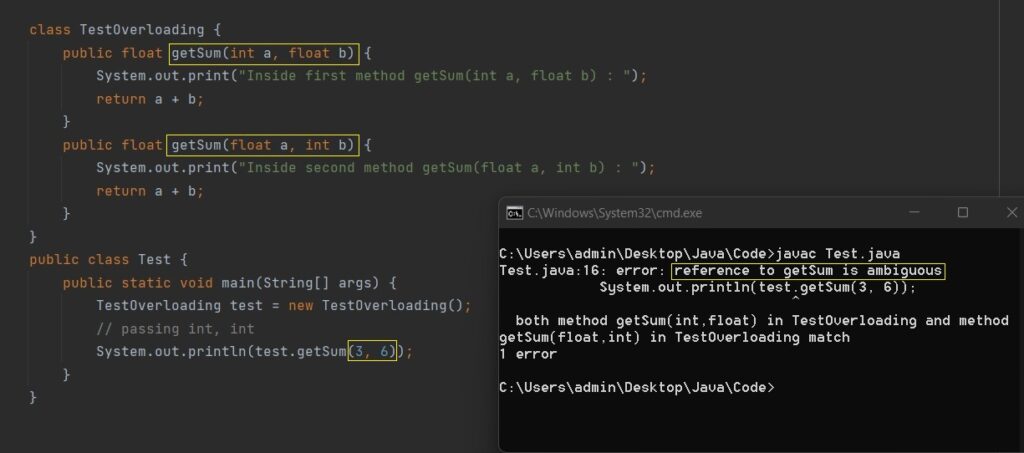
Can we overload/override static methods?
• Overloading: We can overload the methods because both the methods will be present in the same class only. So for overloading static methods is possible in java.
• Overriding: No, we can not override the static methods. Even if parent class and child class have the same static methods then this is not method overriding it’s called Method Hiding.
So the answer is we can not override the static methods, but if we added the same static methods in parent and child then the compiler will not give any error. But this case is called Method hiding instead of overriding.