What is Spring Boot Code Structure?
There is no specific Spring Boot Code Structure but there are some best practices that we as a developer can follow.
We can create multiple packages in our project based on the requirements. If a class does not have any then it is considered as a default package. note that generally default package declaration is not recommended. Spring Boot will cause issues such as auto-configuration or component scan when using the default package.
We have already seen how we can create our project using Spring initializer. The main class package will be generated based on the group name followed by the artifact name. So if our group name is ‘com.ugtworld’ and the artifact name is ‘springbootdemo’ then the package will be like ‘com.ugtworld.springbootdemo’.
shown below.
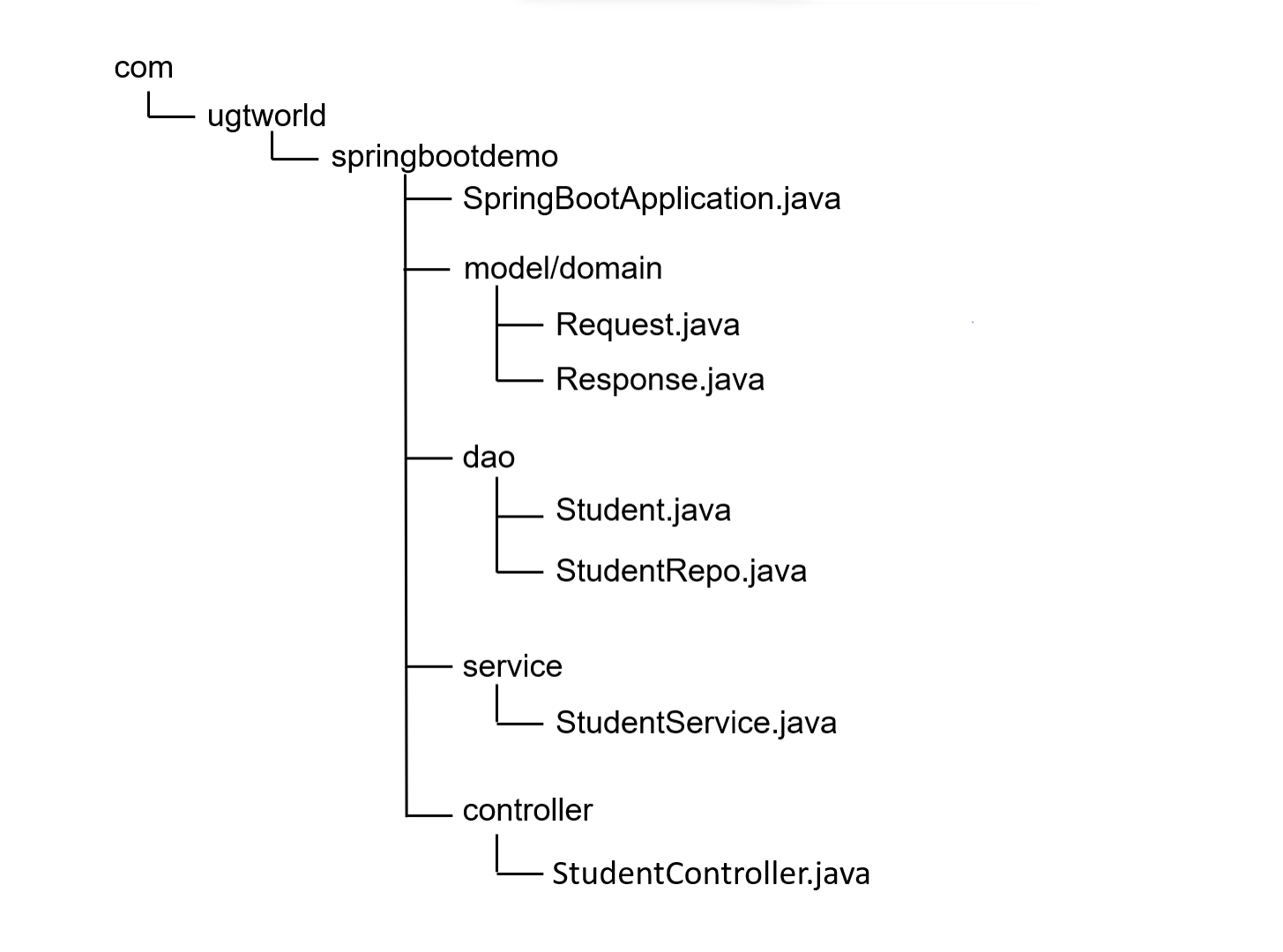
SpringBootApplication.java will have the main class along with @SpringBootApplication annotation as shown below.
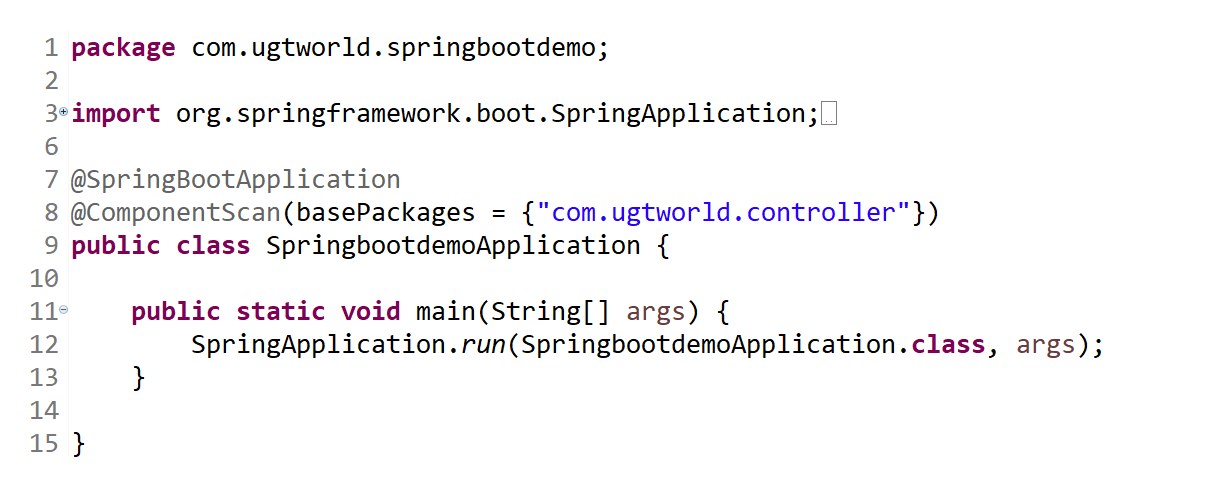
The model or domain package will have POJO / DTO classes of Java like Request.java and Response.java as shown.
Request.java
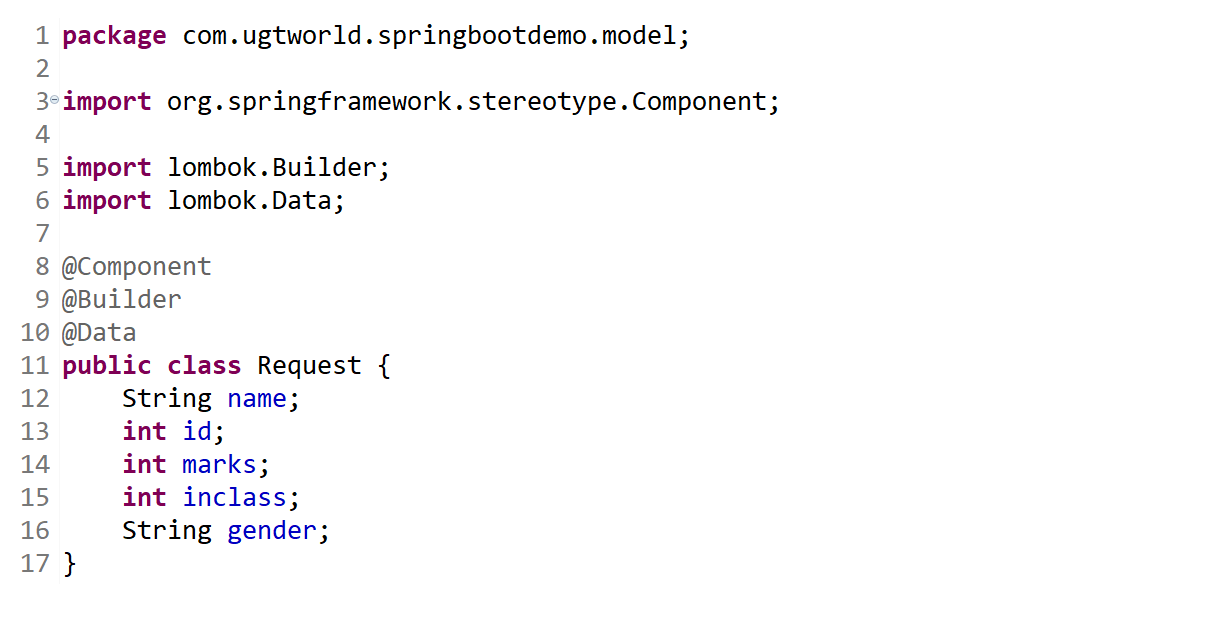
The dao package can have database entity objects for db tables or database repositories as shown below images.
Student.java entity is as shown in the below image.
- As we can see in the image @Entity annotation is used to define the entity class.
- @Table annotation specifies the name of the database table to be used for mapping.
- The @Id annotation specifies the primary key of an entity and the @Column specifies the column name of the table used for mapping.
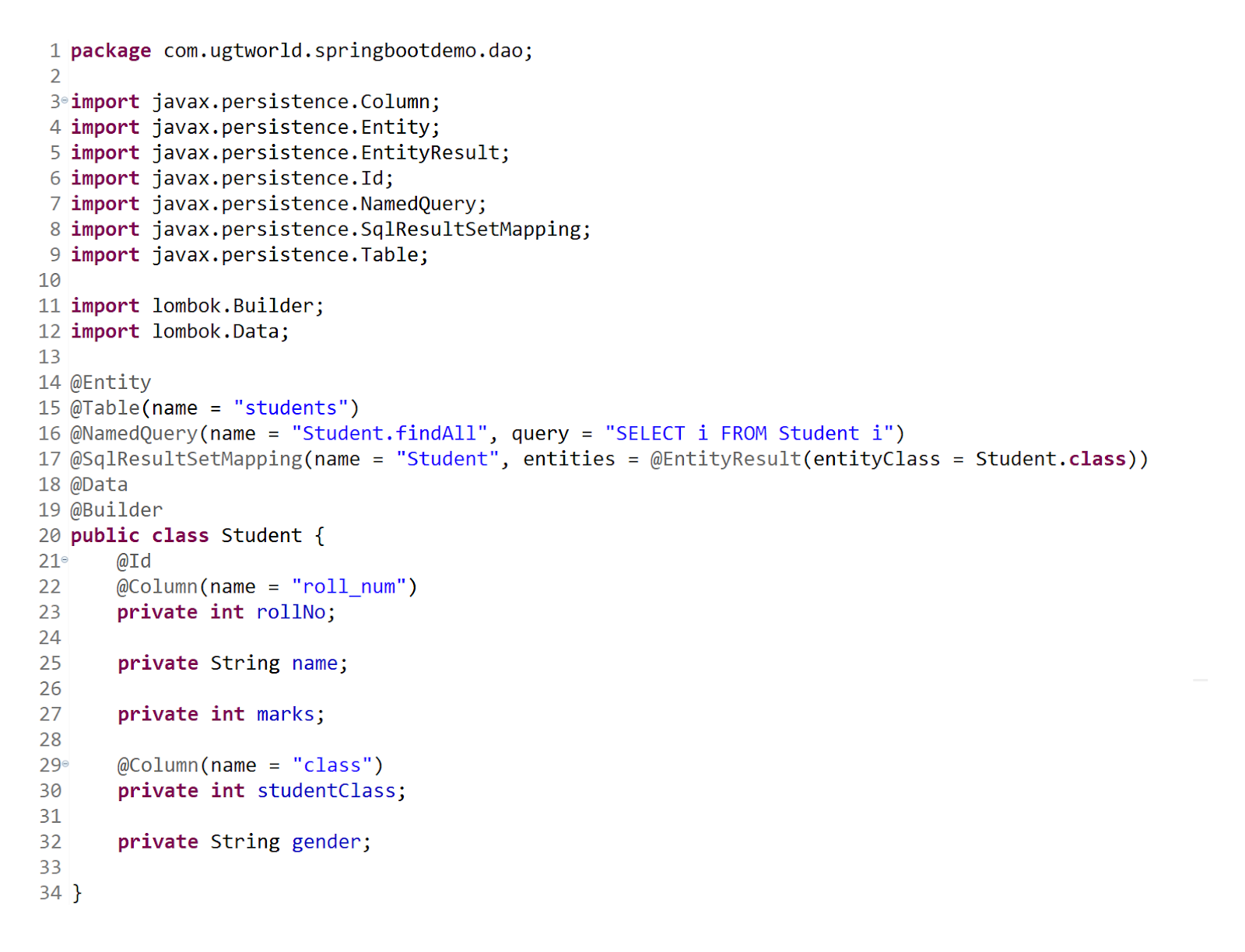
The repository interface StudentRepo.java is shown below.
As we can see from the image. @Repository annotation is used to map the repository interface which extends the JpaRepository interface.
Once entity and repository classes are created, we can call the controller StudentController.java to fetch the student details and write the business logic inside the service class StudentService.java as shown in the below image.
StudentController.java
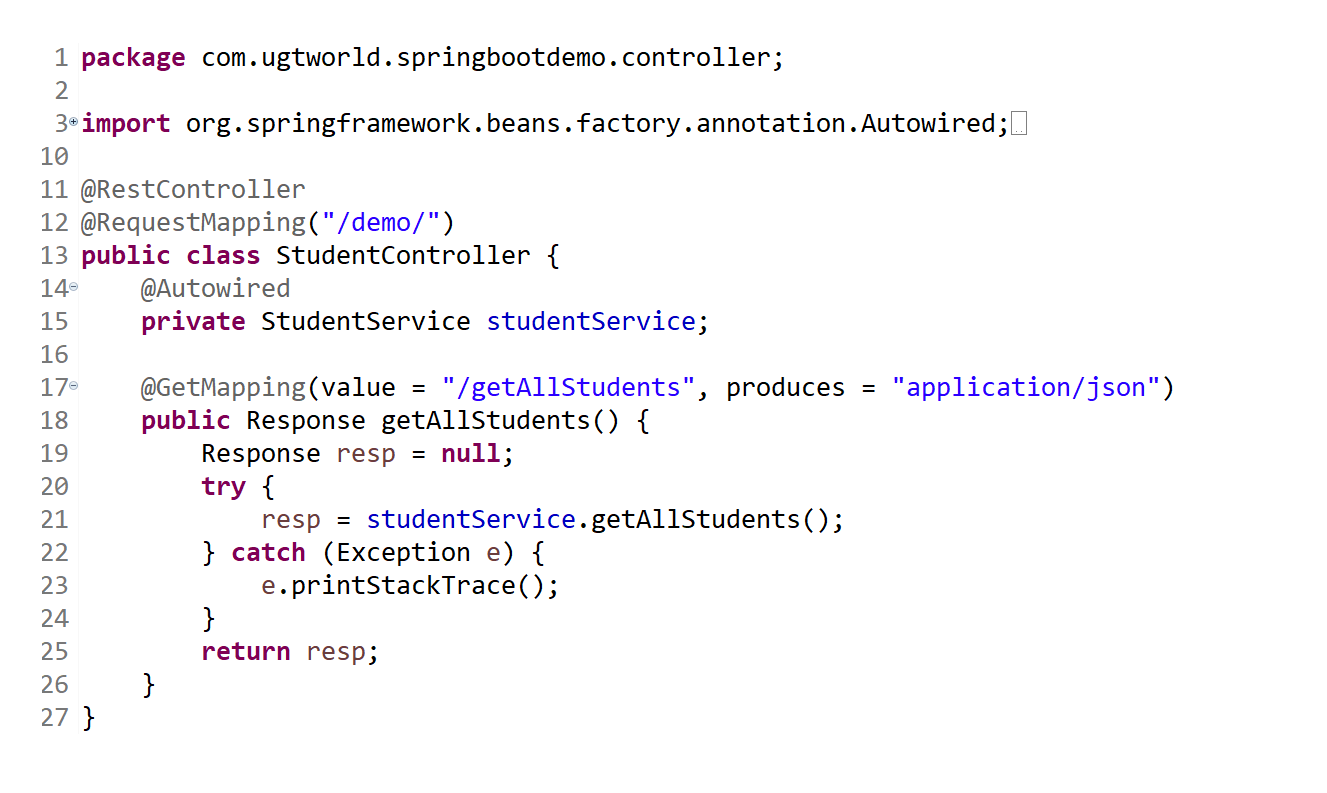
StudentService.java
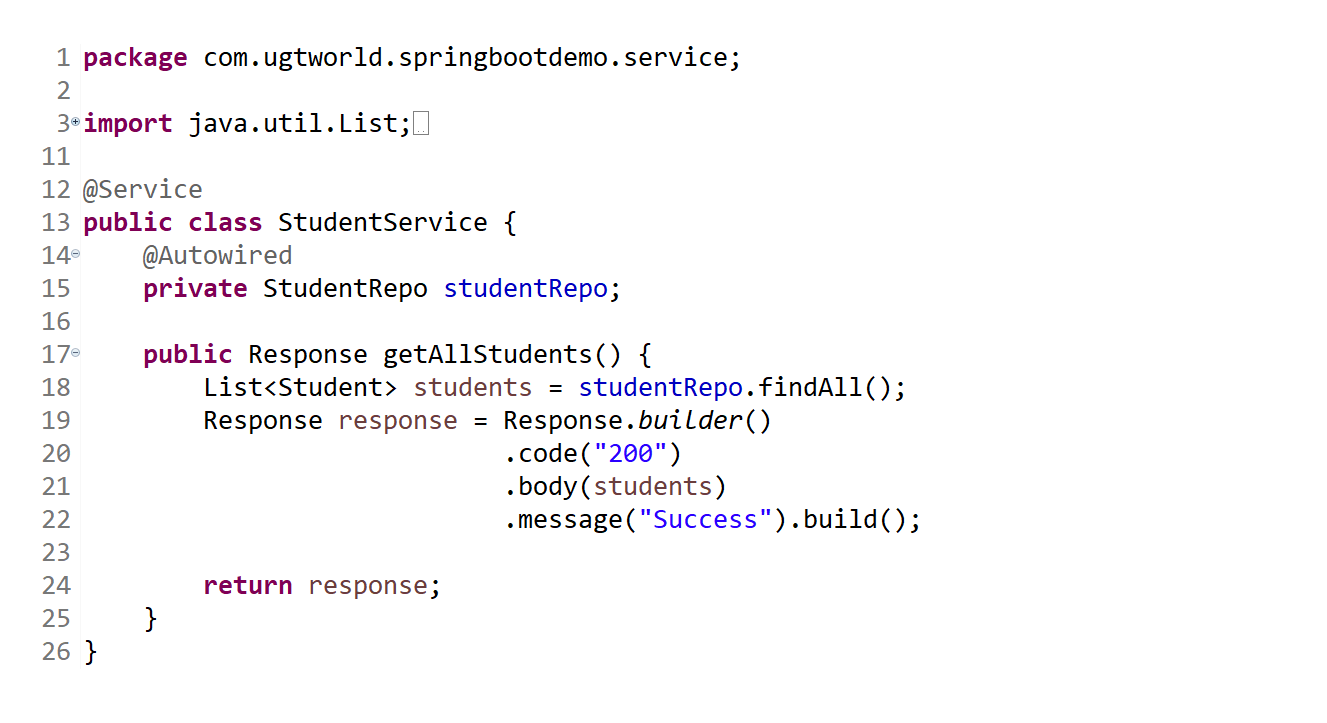
Complete SpringBootProject can be referred from here…