What is Thread Group in Java?
A group of threads based on functionality into a single unit is called a Thread group in java.
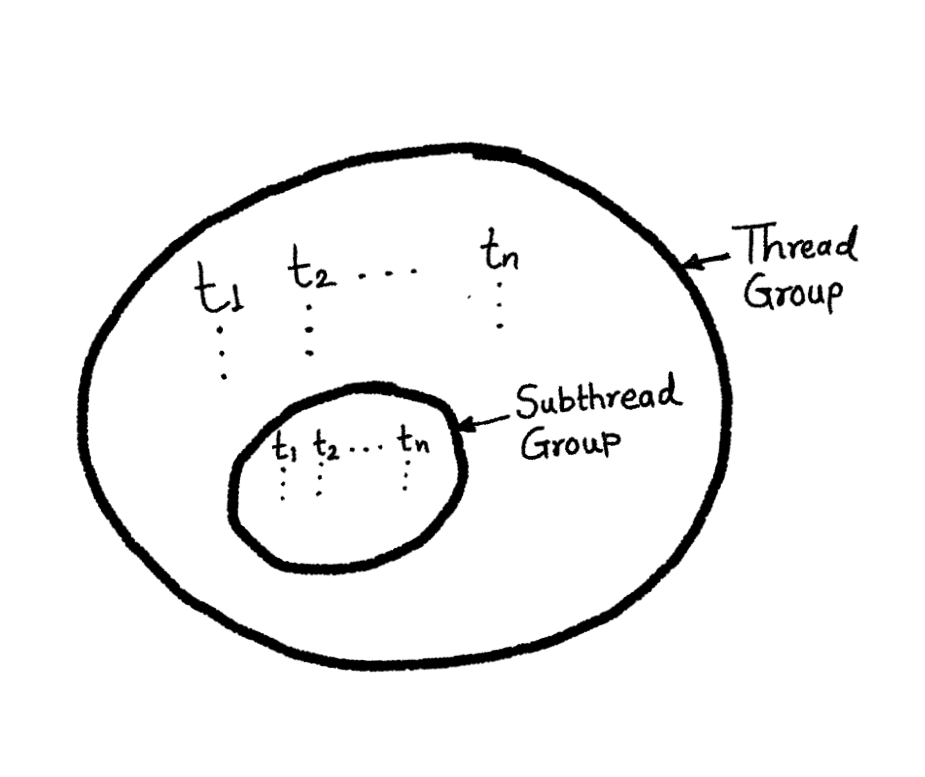
- ThreadGroup is a java class present in java.lang package & It is a direct child class of object.
- Thread groups can also contain subthread groups.
- Advantage: we can perform common operations very easily.
- Every thread in java belongs to some group, main thread belongs to the “main” group.
- Every thread group in java is the child group of the “system” group either directly or indirectly. Hence ‘system’ group acts as a root for all thread groups in java.
- The system group contains several system-level threads like finalizer, Reference Handler, and Signal Dispatcher.
Constructors:
- Thread Group (String name): Creates a new thread group with the specified group name. The parent of this new group is currently executing the thread.
- ThreadGroup (ThreadGroup parent, String name): Creates a new thread group with the specified group name. The parent of this new group is the specified thread in the parameter.
- We can get the name of the thread group by using getThreadGroup().getName() method.
- To get the name of the parent thread group getThreadGroup().getParent().getName().
Example for Thread group in java:
class Test {
public static void main(String args[]) throws InterruptedException {
// Using Thread Group (String name)
ThreadGroup group1 = new ThreadGroup(“Group1”);
MyThread t1 = new MyThread(group1, “thread1”);
// Using Thread Group (ThreadGroup parent, String name)
ThreadGroup childgroup = new ThreadGroup(group1, “ChildGroup”);
MyThread t2 = new MyThread(childgroup, “thread2”);
System.out.println(“Thread name: ” + Thread.currentThread().getName() + “, Group name: “
+ Thread.currentThread().getThreadGroup().getName() + “, Parent group: “
+ Thread.currentThread().getThreadGroup().getParent().getName());
System.out.println(“Thread name: ” + t1.getName() + “, Group name: ” + t1.getThreadGroup().getName()
+ “, Parent group: ” + t1.getThreadGroup().getParent().getName());
System.out.println(“Thread name: ” + t2.getName() + “, Group name: ” + t2.getThreadGroup().getName()
+ “, Parent group: ” + t2.getThreadGroup().getParent().getName());
}
}
class MyThread extends Thread {
MyThread(ThreadGroup group, String name) {
super(group, name);
}
public void run() {
for (int i = 0; i < 5; i++) {
System.out.println(“Running MyThread ” + i);
}
}
}
public static void main(String args[]) throws InterruptedException {
// Using Thread Group (String name)
ThreadGroup group1 = new ThreadGroup(“Group1”);
MyThread t1 = new MyThread(group1, “thread1”);
// Using Thread Group (ThreadGroup parent, String name)
ThreadGroup childgroup = new ThreadGroup(group1, “ChildGroup”);
MyThread t2 = new MyThread(childgroup, “thread2”);
System.out.println(“Thread name: ” + Thread.currentThread().getName() + “, Group name: “
+ Thread.currentThread().getThreadGroup().getName() + “, Parent group: “
+ Thread.currentThread().getThreadGroup().getParent().getName());
System.out.println(“Thread name: ” + t1.getName() + “, Group name: ” + t1.getThreadGroup().getName()
+ “, Parent group: ” + t1.getThreadGroup().getParent().getName());
System.out.println(“Thread name: ” + t2.getName() + “, Group name: ” + t2.getThreadGroup().getName()
+ “, Parent group: ” + t2.getThreadGroup().getParent().getName());
}
}
class MyThread extends Thread {
MyThread(ThreadGroup group, String name) {
super(group, name);
}
public void run() {
for (int i = 0; i < 5; i++) {
System.out.println(“Running MyThread ” + i);
}
}
}
Output:
Thread name: main, Group name: main, Parent group: systemThread name: thread1, Group name: Group1, Parent group: main
Thread name: thread2, Group name: ChildGroup, Parent group: Group1
- From the example we can see that main thread is part of the group “main” and parent group as “system”.
- For thread1 since we did not set any parent group, by default “main” group becomes the parent group for the thread1.